Table Of Contents
Category
Blockchain
Web3
ARVR
AIML
IoT
1. Introduction to Arbitrum and Token Deployment
Arbitrum is a Layer 2 scaling solution for Ethereum that enhances transaction speed and reduces costs while maintaining the security of the Ethereum network. It utilizes optimistic rollups, which allow for off-chain processing of transactions, significantly improving throughput. Deploying tokens on Arbitrum can be advantageous for developers and businesses looking to leverage Ethereum's ecosystem without incurring high gas fees.
1.1. What is Arbitrum and why deploy tokens on it?
Arbitrum is designed to address Ethereum's scalability issues, making it an attractive option for deploying tokens. By using Arbitrum, developers can benefit from:
- Lower transaction fees compared to the Ethereum mainnet.
- Faster transaction confirmations, enhancing user experience.
- Compatibility with existing Ethereum smart contracts, allowing for easy migration.
Deploying tokens on Arbitrum can be particularly beneficial for projects that require high transaction volumes, such as decentralized finance (DeFi) applications and non-fungible tokens (NFTs). The ability to interact with Ethereum's vast ecosystem while enjoying the advantages of a Layer 2 solution makes Arbitrum a compelling choice for token deployment.
At Rapid Innovation, we specialize in guiding businesses through the token deployment process on Arbitrum, ensuring that they maximize their return on investment (ROI) by leveraging our expertise in blockchain technology. Our team can assist in optimizing smart contracts for performance and cost-efficiency, ultimately leading to a more successful project launch.
To successfully deploy your own token on Arbitrum, follow these steps:
- Set up your development environment:
- Install Node.js and npm (Node Package Manager).
- Install Truffle or Hardhat, popular frameworks for Ethereum development.
- Create a new project:
- Initialize a new project directory.
- Run
npm init
to create a package.json file.
- Install necessary dependencies:
- Install OpenZeppelin contracts for ERC20 token standards:
language="language-bash"npm install @openzeppelin/contracts
- Write your token contract:
- Create a new Solidity file (e.g., MyToken.sol).
- Import the ERC20 contract from OpenZeppelin:
language="language-solidity"pragma solidity ^0.8.0;-a1b2c3--a1b2c3- import "@openzeppelin/contracts/token/ERC20/ERC20.sol";-a1b2c3--a1b2c3- contract MyToken is ERC20 {-a1b2c3- constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {-a1b2c3- _mint(msg.sender, initialSupply);-a1b2c3- }-a1b2c3- }
- Configure your deployment script:
- Create a migration file in the migrations directory (e.g., 1deploymytoken.js).
- Add the following code to deploy your token:
language="language-javascript"const MyToken = artifacts.require("MyToken");-a1b2c3--a1b2c3- module.exports = function (deployer) {-a1b2c3- deployer.deploy(MyToken, 1000000); // Initial supply-a1b2c3- };
- Connect to Arbitrum:
- Update your Truffle or Hardhat configuration to include Arbitrum's network settings.
- For Truffle, modify truffle-config.js:
language="language-javascript"networks: {-a1b2c3- arbitrum: {-a1b2c3- provider: () => new HDWalletProvider(mnemonic, `https://arb1.arbitrum.io/rpc`),-a1b2c3- network_id: 42161, // Arbitrum's network ID-a1b2c3- gas: 8000000,-a1b2c3- gasPrice: 20000000000, // 20 gwei-a1b2c3- },-a1b2c3- },
- Deploy your token:
- Run the deployment command:
language="language-bash"truffle migrate --network arbitrum
- Verify your token on Arbitrum:
- Use Etherscan or Arbiscan to verify your token contract.
- Ensure that your token is listed and accessible for trading.
By following these steps, you can successfully deploy your own token on Arbitrum, taking advantage of its scalability and cost-effectiveness. This process opens up new opportunities for developers and businesses in the rapidly evolving blockchain landscape. At Rapid Innovation, we are committed to helping you navigate this landscape effectively, ensuring that your projects achieve their business goals efficiently and effectively. For more information on our services, visit Rapid Innovation.
1.2. Benefits of deploying tokens on Arbitrum

Deploying tokens on Arbitrum offers several advantages that can significantly enhance the performance and usability of decentralized applications (dApps). Here are some key benefits:
- Scalability: Arbitrum utilizes Layer 2 scaling solutions, which allow for a higher throughput of transactions compared to Ethereum's mainnet. This means that deploying tokens on Arbitrum can handle more users and transactions without congestion, ultimately leading to improved user satisfaction and retention.
- Lower Transaction Fees: One of the most appealing aspects of Arbitrum is its reduced gas fees. Deploying tokens on Arbitrum can save users money, making it more attractive for small transactions and microtransactions. This cost-effectiveness can lead to increased transaction volume and, consequently, greater revenue potential for businesses.
- Faster Transactions: Transactions on Arbitrum are processed much quicker than on the Ethereum mainnet. This speed enhances user experience, especially for applications that require real-time interactions, thereby increasing user engagement and loyalty.
- Interoperability: Arbitrum is designed to be compatible with Ethereum, allowing developers to leverage existing Ethereum tools and libraries. This makes it easier to migrate or deploy new tokens on Arbitrum without extensive modifications, reducing development time and costs.
- Security: Arbitrum inherits the security features of Ethereum, ensuring that your tokens are secure while benefiting from the enhanced performance of Layer 2 solutions. This security assurance can build trust with users and stakeholders, which is crucial for long-term success.
- Developer-Friendly: The development environment on Arbitrum is similar to Ethereum, making it easier for developers to transition and build applications. This familiarity can lead to faster development cycles and reduced learning curves, allowing businesses to bring their products to market more quickly.
1.3. Prerequisites for this tutorial
Before diving into the tutorial on deploying tokens on Arbitrum, ensure you have the following prerequisites:
- Basic Knowledge of Smart Contracts: Familiarity with Solidity and the Ethereum Virtual Machine (EVM) is essential for understanding how to create and deploy tokens.
- Node.js and npm Installed: Ensure you have Node.js and npm (Node Package Manager) installed on your machine. These tools are necessary for managing packages and running scripts.
- Metamask Wallet: A Metamask wallet is required to interact with the Ethereum network and Arbitrum. Make sure you have it installed and set up with some ETH for transaction fees.
- Arbitrum Network Configuration: You need to configure your Metamask to connect to the Arbitrum network. This involves adding the Arbitrum network details to your wallet.
- Development Environment: A code editor (like Visual Studio Code) and a terminal for running commands are recommended for a smooth development experience.
2. Setting Up Your Development Environment
Setting up your development environment is crucial for deploying tokens on Arbitrum. Follow these steps to ensure everything is ready:
- Install Node.js and npm:
- Download and install Node.js from the official website.
- Verify the installation by running the following commands in your terminal:
language="language-bash"node -v-a1b2c3- npm -v
- Install Truffle or Hardhat:
- Choose a development framework like Truffle or Hardhat. For example, to install Hardhat, run:
language="language-bash"npm install --save-dev hardhat
- Create a New Project:
- Create a new directory for your project and navigate into it:
language="language-bash"mkdir my-arbitrum-token-a1b2c3- cd my-arbitrum-token
- Initialize the Project:
- Initialize your project with Hardhat:
language="language-bash"npx hardhat
- Install Required Dependencies:
- Install OpenZeppelin contracts for token standards:
language="language-bash"npm install @openzeppelin/contracts
- Configure Metamask:
- Open Metamask and add the Arbitrum network by entering the following details:
- Network Name: Arbitrum
- New RPC URL: https://arb1.arbitrum.io/rpc
- Chain ID: 42161
- Currency Symbol: ETH
- Open Metamask and add the Arbitrum network by entering the following details:
- Connect to Arbitrum:
- Ensure your Metamask wallet is connected to the Arbitrum network and has some ETH for gas fees.
By following these steps, you will have a fully functional development environment ready for deploying tokens on Arbitrum.
2.1. Installing necessary tools (Node.js, npm, Hardhat)
To start developing on the Ethereum blockchain and deploy smart contracts, you need to install several essential tools. The primary tools include Node.js, npm (Node Package Manager), and Hardhat.
- Node.js: This is a JavaScript runtime that allows you to run JavaScript code server-side. It is crucial for running Hardhat and other development tools.
- npm: This is a package manager for JavaScript that comes bundled with Node.js. It allows you to install libraries and tools needed for your project.
- Hardhat: This is a development environment specifically designed for Ethereum. It helps in compiling, deploying, testing, and debugging smart contracts.
To install these tools, follow these steps:
- Download and install Node.js from the official website.
- Verify the installation by running the following commands in your terminal:
language="language-bash"node -v-a1b2c3- npm -v
- Install Hardhat globally using npm:
language="language-bash"npm install --global hardhat
For more detailed guidance on building decentralized applications, you can refer to this guide on building a DApp on Aptos blockchain.
2.2. Creating a new Hardhat project
Once you have installed the necessary tools, you can create a new Hardhat project. This process sets up a directory structure and configuration files that are essential for your development.
- Open your terminal and create a new directory for your project:
language="language-bash"mkdir my-hardhat-project-a1b2c3- cd my-hardhat-project
- Initialize a new npm project:
language="language-bash"npm init -y
- Install Hardhat in your project:
language="language-bash"npm install --save-dev hardhat
- Create a new Hardhat project by running:
language="language-bash"npx hardhat
- Follow the prompts to set up your project. You can choose to create a sample project, which includes example contracts and tests.
2.3. Configuring Hardhat for Arbitrum deployment
If you plan to deploy your smart contracts on the Arbitrum network, you need to configure Hardhat accordingly. Arbitrum is a Layer 2 scaling solution for Ethereum, and it requires specific settings in your Hardhat configuration file.
- Open the
hardhat.config.js
file in your project directory. - Add the following configuration to set up the Arbitrum network:
language="language-javascript"require('@nomiclabs/hardhat-waffle');-a1b2c3--a1b2c3- module.exports = {-a1b2c3- solidity: "0.8.4",-a1b2c3- networks: {-a1b2c3- arbitrum: {-a1b2c3- url: 'https://arb1.arbitrum.io/rpc',-a1b2c3- accounts: ['YOUR_PRIVATE_KEY']-a1b2c3- }-a1b2c3- }-a1b2c3- };
- Replace
YOUR_PRIVATE_KEY
with the private key of the wallet you will use for deployment. Ensure you keep this key secure and do not expose it in public repositories. - Install the necessary dependencies for Arbitrum:
language="language-bash"npm install --save-dev @nomiclabs/hardhat-ethers ethers
By following these steps, you will have a fully set up Hardhat environment ready for Ethereum and Arbitrum development. This setup allows you to compile, test, and deploy your smart contracts efficiently.
At Rapid Innovation, we understand that the deployment of smart contracts is just the beginning. Our team of experts can guide you through the entire development lifecycle, ensuring that your blockchain solutions are not only robust but also aligned with your business objectives. By leveraging our expertise in AI and blockchain, we help clients achieve greater ROI through optimized processes and innovative solutions tailored to their specific needs. For more information on our services, check out our Blockchain as a Service.
3. Writing Your Token Smart Contract
3.1. Understanding ERC-20 Token Standard
The ERC-20 token standard is a widely adopted protocol for creating fungible tokens on the Ethereum blockchain. It defines a set of rules and functions that a token contract must implement, ensuring interoperability between different tokens and applications. Understanding this standard is crucial for anyone looking to create their own erc20 token creation.
Key features of the ERC-20 standard include:
- Fungibility: Each token is identical and interchangeable with another token of the same type.
- Transferability: Tokens can be easily transferred between users.
- Approval Mechanism: Users can approve third-party contracts to spend tokens on their behalf.
- Events: The standard includes events like
Transfer
andApproval
to notify external applications of token movements.
The ERC-20 standard includes six mandatory functions:
totalSupply()
: Returns the total supply of tokens.balanceOf(address _owner)
: Returns the balance of a specific address.transfer(address _to, uint256 _value)
: Transfers tokens to a specified address.transferFrom(address _from, address _to, uint256 _value)
: Allows a user to transfer tokens from one address to another.approve(address _spender, uint256 _value)
: Approves a third-party address to spend tokens on behalf of the user.allowance(address _owner, address _spender)
: Returns the amount of tokens that a spender is allowed to withdraw from the owner's account.
Understanding the ERC-20 standard is essential for businesses looking to leverage blockchain technology for tokenization, as it provides a foundation for creating digital assets that can enhance liquidity and facilitate transactions.
3.2. Implementing the Token Contract in Solidity
Once you understand the ERC-20 standard, the next step is to implement your token contract using Solidity, the programming language for Ethereum smart contracts. Below are the steps to create a basic erc 20 token creation contract.
- Set up your development environment: Use tools like Remix IDE or Truffle to write and deploy your smart contract.
- Create the contract: Start by defining your contract and importing the OpenZeppelin library, which provides secure implementations of ERC-20.
language="language-solidity"// SPDX-License-Identifier: MIT-a1b2c3-pragma solidity ^0.8.0;-a1b2c3--a1b2c3-import "@openzeppelin/contracts/token/ERC20/ERC20.sol";-a1b2c3--a1b2c3-contract MyToken is ERC20 {-a1b2c3- constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {-a1b2c3- _mint(msg.sender, initialSupply);-a1b2c3- }-a1b2c3-}
- Define the constructor: The constructor initializes the token with a name and symbol, and mints an initial supply of tokens to the contract creator.
- Deploy the contract: Use Remix or Truffle to deploy your contract to the Ethereum network. Make sure to have some Ether in your wallet to cover gas fees.
- Interact with the contract: After deployment, you can interact with your token using functions like
transfer
,approve
, andtransferFrom
. - Test your contract: Always test your contract on a test network (like Ropsten or Rinkeby) before deploying it on the main Ethereum network.
By following these steps, you can successfully create and deploy your own erc20 token creation service on the Ethereum blockchain. This process not only enhances your understanding of smart contracts but also opens up opportunities for various applications in decentralized finance (DeFi) and beyond. At Rapid Innovation, we specialize in guiding businesses through this process, ensuring that your token implementation aligns with your strategic goals and maximizes your return on investment. Our expertise in blockchain development can help you navigate the complexities of token creation, enabling you to leverage the full potential of blockchain technology for your business.
3.3. Adding Custom Functionality to Your Token
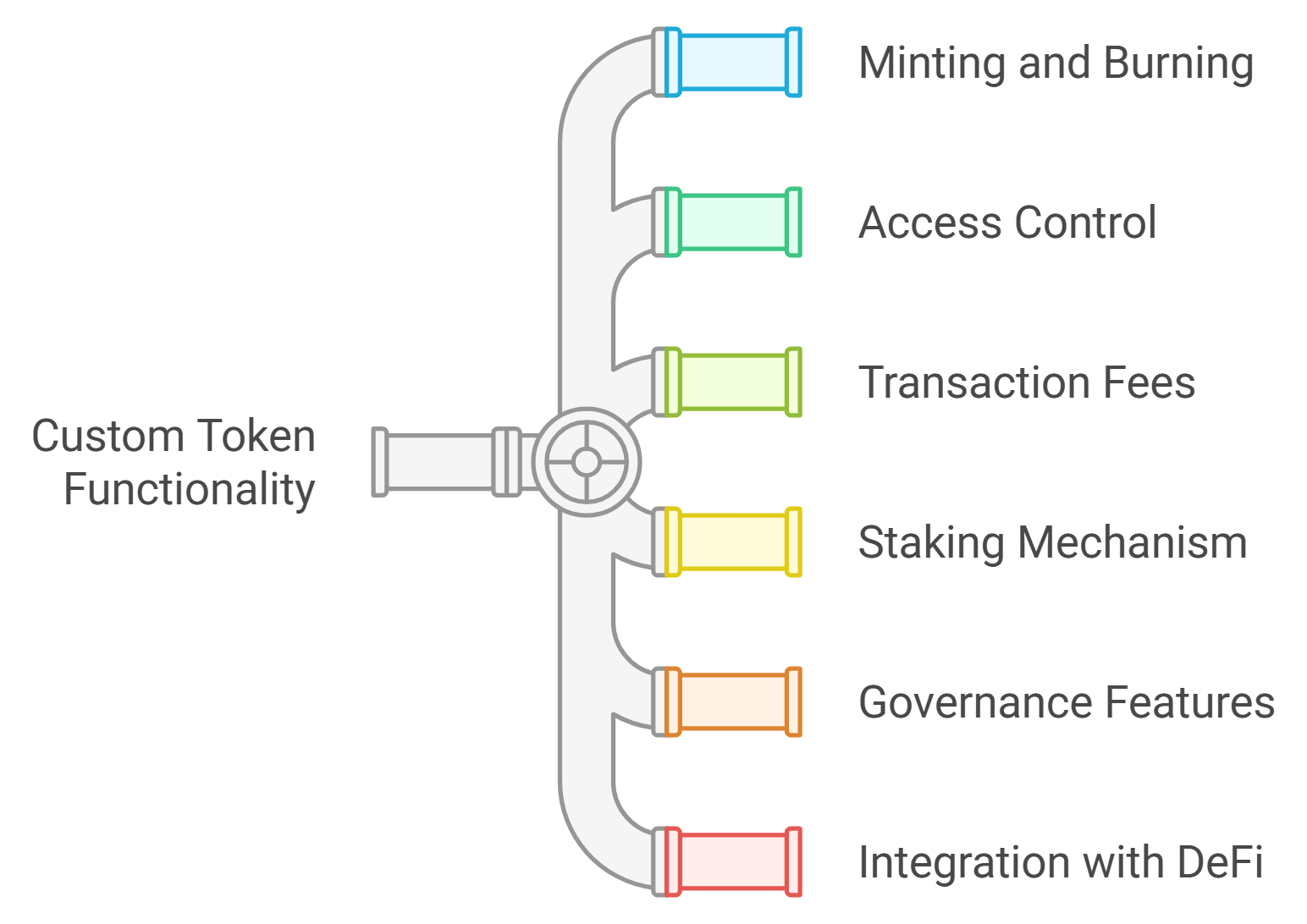
When creating a token, you may want to add custom functionality to enhance its utility and appeal. Token development features can differentiate your token from others and provide additional value to users. Here are some common functionalities you might consider:
- Minting and Burning: Allow users to create (mint) or destroy (burn) tokens. This can help manage supply and demand effectively.
- Access Control: Implement role-based access control to restrict certain functions to specific users or roles. This can enhance security and governance.
- Transaction Fees: Introduce a fee for transactions that can be redistributed to holders or used for development purposes. This can incentivize holding and create a sustainable ecosystem.
- Staking Mechanism: Allow users to stake their tokens to earn rewards. This can encourage long-term holding and increase token value.
- Governance Features: Enable token holders to vote on important decisions regarding the project. This can foster community engagement and decentralization.
- Integration with Decentralized Finance (DeFi): Consider integrating your token with DeFi platforms for lending, borrowing, or liquidity provision. This can enhance the token's utility.
To implement these functionalities, you will typically modify the smart contract code. Here’s a basic outline of steps to add custom functionality:
- Identify the desired functionality.
- Modify the smart contract code in Solidity.
- Test the new features locally.
- Deploy the updated contract to a test network.
- Conduct thorough testing to ensure everything works as intended.
4. Compiling and Testing Your Token Contract
Once you have added custom functionality to your token, the next step is to compile and test your token contract. This process ensures that your code is free of errors and behaves as expected.
- Compiling: Use a Solidity compiler to convert your smart contract code into bytecode that can be executed on the Ethereum Virtual Machine (EVM). This step is crucial as it checks for syntax errors and other issues.
- Testing: After compiling, you should rigorously test your contract. This includes unit tests for individual functions and integration tests to ensure that all components work together seamlessly.
- Tools for Testing: Utilize frameworks like Truffle or Hardhat, which provide built-in testing environments and tools to facilitate the testing process.
- Test Coverage: Aim for high test coverage to ensure that all parts of your contract are tested. This can help identify potential vulnerabilities or bugs.
4.1. Compiling the Solidity Contract
Compiling your Solidity contract is a critical step in the development process. Here’s how to do it effectively:
- Set Up Your Environment: Ensure you have the necessary tools installed, such as Node.js, npm, and a Solidity compiler like solc.
- Write Your Contract: Create your Solidity contract file (e.g., MyToken.sol) and include all necessary functionalities.
- Use a Compiler: You can compile your contract using the command line or an integrated development environment (IDE) like Remix.
- Command Line Compilation:
- Open your terminal.
- Navigate to the directory containing your contract.
- Run the command:
language="language-bash"solc --bin --abi MyToken.sol -o output/
- This command generates the bytecode and ABI (Application Binary Interface) for your contract.
- Check for Errors: Review the output for any compilation errors or warnings. Address these issues before proceeding.
- Deploy to Test Network: Once compiled successfully, deploy your contract to a test network (like Rinkeby or Ropsten) to further test its functionality in a live environment.
By following these steps, you can ensure that your token contract is robust, functional, and ready for deployment.
At Rapid Innovation, we specialize in guiding clients through the entire token development process, from conceptualization to deployment. Our expertise in blockchain technology ensures that your token not only meets your business objectives but also stands out in a competitive market, ultimately leading to greater ROI. For more information on how we can assist you with smart contract development, visit our Smart Contract Development page.
4.2. Writing unit tests for your token
Unit testing is a crucial step in the development of smart contracts, including tokens. It ensures that your token behaves as expected under various conditions. Writing unit tests for your token can help catch bugs early and improve the overall reliability of your code.
To write unit tests for your token, follow these steps:
- Set up your testing environment using Hardhat.
- Create a new test file in the
test
directory of your Hardhat project. - Import necessary libraries, such as
chai
for assertions andethers
for interacting with your smart contract. - Write test cases for each function in your token contract. Common functions to test include:
transfer
approve
transferFrom
balanceOf
- Use assertions to verify that the expected outcomes match the actual outcomes.
Example code snippet for a simple token transfer test:
language="language-javascript"const { expect } = require("chai");-a1b2c3--a1b2c3-describe("Token Contract", function () {-a1b2c3- let Token;-a1b2c3- let token;-a1b2c3- let owner;-a1b2c3- let addr1;-a1b2c3--a1b2c3- beforeEach(async function () {-a1b2c3- Token = await ethers.getContractFactory("Token");-a1b2c3- [owner, addr1] = await ethers.getSigners();-a1b2c3- token = await Token.deploy();-a1b2c3- await token.deployed();-a1b2c3- });-a1b2c3--a1b2c3- it("Should transfer tokens between accounts", async function () {-a1b2c3- await token.transfer(addr1.address, 50);-a1b2c3- const addr1Balance = await token.balanceOf(addr1.address);-a1b2c3- expect(addr1Balance).to.equal(50);-a1b2c3- });-a1b2c3-});
4.3. Running and debugging tests in Hardhat
Once you have written your unit tests, the next step is to run and debug them using Hardhat. Hardhat provides a robust testing framework that allows you to execute your tests efficiently.
To run and debug your tests, follow these steps:
- Open your terminal and navigate to your Hardhat project directory.
- Run the following command to execute your tests:
language="language-bash"npx hardhat test
- If you encounter any errors, Hardhat will provide detailed output, including the line number and error message, making it easier to debug.
- You can also use the
--network
flag to specify a network if you are testing on a specific one.
For debugging, you can use the Hardhat console:
- Start the Hardhat console with:
language="language-bash"npx hardhat console
- You can interactively test your contract functions and check the state of your contract.
5. Deploying Your Token to Arbitrum Testnet
Deploying your token to the Arbitrum Testnet allows you to test your token in a live environment without spending real Ether. Arbitrum is a Layer 2 scaling solution for Ethereum, providing faster and cheaper transactions.
To deploy your token to the Arbitrum Testnet, follow these steps:
- Ensure you have an Arbitrum Testnet wallet set up with some test Ether.
- Update your Hardhat configuration file (
hardhat.config.js
) to include the Arbitrum Testnet settings:
language="language-javascript"module.exports = {-a1b2c3- networks: {-a1b2c3- arbitrumTestnet: {-a1b2c3- url: "https://rinkeby.arbitrum.io/rpc",-a1b2c3- accounts: [`0x${YOUR_PRIVATE_KEY}`],-a1b2c3- },-a1b2c3- },-a1b2c3-};
- Create a deployment script in the
scripts
directory. For example,deploy.js
:
language="language-javascript"async function main() {-a1b2c3- const Token = await ethers.getContractFactory("Token");-a1b2c3- const token = await Token.deploy();-a1b2c3- await token.deployed();-a1b2c3- console.log("Token deployed to:", token.address);-a1b2c3-}-a1b2c3--a1b2c3-main()-a1b2c3- .then(() => process.exit(0))-a1b2c3- .catch((error) => {-a1b2c3- console.error(error);-a1b2c3- process.exit(1);-a1b2c3- });
- Run the deployment script using the following command:
language="language-bash"npx hardhat run scripts/deploy.js --network arbitrumTestnet
- After successful deployment, you will see the contract address in the console output, confirming that your token is now live on the Arbitrum Testnet.
At Rapid Innovation, we understand the importance of robust token unit testing and deployment processes in achieving your business goals. Our expertise in AI and Blockchain development ensures that your smart contracts are not only functional but also secure and efficient, ultimately leading to greater ROI for your projects. By leveraging our services, you can focus on your core business while we handle the complexities of blockchain technology.
5.1. Setting up an Arbitrum testnet wallet
To interact with the Arbitrum testnet, you need a compatible wallet. The most commonly used wallets are MetaMask and WalletConnect. Here’s how to set up a wallet for the Arbitrum testnet:
- Download and install MetaMask from the official website.
- Create a new wallet or import an existing one using your seed phrase.
- Once your wallet is set up, switch to the Arbitrum testnet:
- Click on the network dropdown at the top of the MetaMask interface.
- Select "Custom RPC."
- Enter the following details:
- Network Name: Arbitrum Testnet
- New RPC URL:
https://rinkeby.arbitrum.io/rpc
- Chain ID: 421611
- Currency Symbol: ETH
- Block Explorer URL:
https://rinkeby-explorer.arbitrum.io/
- Save the settings and switch to the Arbitrum Testnet.
5.2. Obtaining testnet ETH from Arbitrum faucet
Once your wallet is set up, you will need testnet ETH to deploy contracts or interact with dApps on the Arbitrum testnet. You can obtain testnet ETH from the Arbitrum faucet. Follow these steps:
- Visit the Arbitrum faucet website (ensure you are on the official site).
- Connect your MetaMask wallet to the faucet.
- Request testnet ETH by entering your wallet address.
- Complete any CAPTCHA or verification steps required by the faucet.
- After submitting your request, you should receive testnet ETH in your wallet shortly.
Note: Faucets may have limits on the amount of ETH you can request, so check the faucet's guidelines.
5.3. Writing the deployment script
If you are deploying a smart contract on the Arbitrum testnet, you will need a deployment script. This script can be written using JavaScript with the Hardhat framework. Here’s how to create a simple deployment script:
- Install Hardhat in your project directory:
language="language-bash"npm install --save-dev hardhat
- Create a new Hardhat project:
language="language-bash"npx hardhat
- Choose "Create a sample project" and follow the prompts.
- Navigate to the
scripts
folder and create a new file nameddeploy.js
. - In
deploy.js
, write the following code to deploy your contract:
language="language-javascript"const hre = require("hardhat");-a1b2c3--a1b2c3- async function main() {-a1b2c3- const Contract = await hre.ethers.getContractFactory("YourContractName");-a1b2c3- const contract = await Contract.deploy();-a1b2c3- await contract.deployed();-a1b2c3- console.log("Contract deployed to:", contract.address);-a1b2c3- }-a1b2c3--a1b2c3- main()-a1b2c3- .then(() => process.exit(0))-a1b2c3- .catch((error) => {-a1b2c3- console.error(error);-a1b2c3- process.exit(1);-a1b2c3- });
- Replace
"YourContractName"
with the name of your smart contract. - To deploy your contract, run the following command in your terminal:
language="language-bash"npx hardhat run scripts/deploy.js --network arbitrumTestnet
By following these steps, you will have set up an Arbitrum testnet wallet, obtained testnet ETH, and written a deployment script to deploy your smart contract on the Arbitrum testnet. This process allows developers to test their applications in a safe environment before going live on the mainnet.
At Rapid Innovation, we specialize in guiding businesses through the complexities of blockchain technology, ensuring that your projects are not only technically sound but also aligned with your strategic goals. By leveraging our expertise in Bitcoin wallet development, you can achieve greater ROI through efficient deployment and testing processes, ultimately accelerating your time to market.
5.4. Executing the deployment on Arbitrum testnet
Deploying your token on the Arbitrum testnet is a crucial step in the development process. Arbitrum is a Layer 2 scaling solution for Ethereum, which allows for faster and cheaper transactions. Here’s how to execute the deployment:
- Set Up Your Environment: Ensure you have Node.js and npm installed. You will also need a wallet like MetaMask configured for the Arbitrum testnet.
- Install Hardhat: Use Hardhat, a development environment for Ethereum software, to facilitate the deployment.
language="language-bash"npm install --save-dev hardhat
- Create a Hardhat Project: Initialize a new Hardhat project.
language="language-bash"npx hardhat
- Configure Network Settings: In your Hardhat configuration file (
hardhat.config.js
), add the Arbitrum testnet settings.
language="language-javascript"require('@nomiclabs/hardhat-waffle');-a1b2c3--a1b2c3- module.exports = {-a1b2c3- solidity: "0.8.0",-a1b2c3- networks: {-a1b2c3- arbitrumTestnet: {-a1b2c3- url: "https://rinkeby.arbitrum.io/rpc",-a1b2c3- accounts: [`0x${YOUR_PRIVATE_KEY}`]-a1b2c3- }-a1b2c3- }-a1b2c3- };
- Write Your Smart Contract: Create a new Solidity file in the
contracts
directory for your token. - Deploy the Contract: Create a deployment script in the
scripts
folder.
language="language-javascript"async function main() {-a1b2c3- const Token = await ethers.getContractFactory("YourToken");-a1b2c3- const token = await Token.deploy();-a1b2c3- await token.deployed();-a1b2c3- console.log("Token deployed to:", token.address);-a1b2c3- }-a1b2c3--a1b2c3- main()-a1b2c3- .then(() => process.exit(0))-a1b2c3- .catch((error) => {-a1b2c3- console.error(error);-a1b2c3- process.exit(1);-a1b2c3- });
- Run the Deployment Script: Execute the deployment script to deploy your token on the Arbitrum testnet.
language="language-bash"npx hardhat run scripts/deploy.js --network arbitrumTestnet
6. Verifying Your Token Contract on Arbiscan
Once your token is deployed, verifying your contract on Arbiscan is essential for transparency and trust. Verification allows users to see the source code of your contract, ensuring it matches the deployed bytecode.
To verify your contract, follow these steps:
- Prepare Your Contract for Verification: Ensure your contract is well-documented and follows best practices.
- Get Your Contract Address: After deployment, note the contract address provided in the console.
- Visit Arbiscan: Go to the Arbiscan website and navigate to the contract verification section.
- Fill Out the Verification Form: Input the necessary details, including:
- Contract Address
- Compiler Version
- Optimization settings
- Source Code
- Submit the Verification Request: After filling out the form, submit your request. Arbiscan will compile your code and check it against the deployed bytecode.
6.1. Understanding contract verification importance
Contract verification is vital for several reasons:
- Transparency: Users can review the source code, ensuring it behaves as expected.
- Trust: Verified contracts build trust within the community, as users can confirm the legitimacy of the token.
- Security: Verification can help identify potential vulnerabilities in the code, allowing for timely fixes.
- Interoperability: Other developers can interact with your contract more easily when it is verified, promoting collaboration and integration.
By following these steps and understanding the importance of contract verification, you can ensure a successful deployment and foster trust in your token project on the Arbitrum testnet.
At Rapid Innovation, we specialize in guiding clients through the complexities of blockchain deployment and verification processes. Our expertise ensures that your token is not only deployed efficiently but also verified to enhance trust and security, ultimately leading to greater ROI for your business. For a more detailed guide, check out our comprehensive guide on how to create custom tokens like ARB.
6.2. Preparing contract metadata for verification
When deploying smart contracts on the Ethereum blockchain, it is crucial to prepare contract metadata for verification. This metadata provides essential information about the contract, enabling users and developers to verify the source code against the deployed bytecode on the blockchain. Proper verification enhances transparency and trust in your smart contract, which is vital for fostering user confidence and ensuring compliance with regulatory standards.
To prepare contract metadata, follow these steps:
- Gather Contract Information: Collect the following details:
- Contract name
- Compiler version
- Optimization settings
- Solidity source code
- Create a Metadata File: This file typically includes:
- The contract's ABI (Application Binary Interface)
- The bytecode of the contract
- The network on which the contract is deployed
- Use a Verification Tool: Tools like Etherscan or Hardhat can help automate the verification process. Ensure you have the correct API key for the verification service. For example, you can use etherscan verified contracts or hardhat verify contract to facilitate this process.
- Compile the Contract: Use the Solidity compiler to generate the ABI and bytecode. This can be done using Hardhat or Truffle.
- Verify the Contract: Submit the metadata to the verification service. This usually involves:
- Inputting the contract address
- Uploading the metadata file
- Confirming the details match the deployed contract
By preparing accurate contract metadata, you ensure that your smart contract can be easily verified, fostering trust among users and developers. At Rapid Innovation, we assist clients in this process, ensuring that their smart contracts are not only deployed efficiently but also verified correctly, which can significantly enhance their return on investment (ROI) by building user trust and reducing the risk of disputes.
6.3. Using Hardhat for automated contract verification
Hardhat is a powerful development environment for Ethereum that simplifies the process of deploying and verifying smart contracts. It provides built-in tools and plugins that streamline the verification process, making it easier for developers to ensure their contracts are trustworthy.
To use Hardhat for automated contract verification, follow these steps:
- Install Hardhat: If you haven't already, install Hardhat in your project directory:
language="language-bash"npm install --save-dev hardhat
- Set Up Hardhat Project: Initialize a new Hardhat project:
language="language-bash"npx hardhat
- Install Verification Plugin: Add the Hardhat Etherscan plugin for verification:
language="language-bash"npm install --save-dev @nomiclabs/hardhat-etherscan
- Configure Hardhat: Update your
hardhat.config.js
file to include your Etherscan API key:
language="language-javascript"require("@nomiclabs/hardhat-etherscan");-a1b2c3--a1b2c3- module.exports = {-a1b2c3- etherscan: {-a1b2c3- apiKey: "YOUR_ETHERSCAN_API_KEY",-a1b2c3- },-a1b2c3- // other configurations-a1b2c3- };
- Compile Your Contracts: Ensure your contracts are compiled:
language="language-bash"npx hardhat compile
- Deploy Your Contracts: Deploy your contracts to the desired network:
language="language-bash"npx hardhat run scripts/deploy.js --network <network_name>
- Verify Your Contracts: Use the Hardhat command to verify your deployed contract:
language="language-bash"npx hardhat verify --network <network_name> <contract_address> <constructor_arguments>
By following these steps, you can automate the verification process, saving time and reducing the potential for human error. Rapid Innovation leverages Hardhat's capabilities to streamline this process for our clients, ensuring that their smart contracts are not only deployed but also verified efficiently, thereby maximizing their operational efficiency and ROI.
7. Interacting with Your Deployed Token
Once your token is deployed and verified, interacting with it becomes essential for users and developers. This interaction can include transferring tokens, checking balances, and executing other functions defined in the smart contract.
To interact with your deployed token, consider the following:
- Use a Web3 Library: Libraries like Web3.js or Ethers.js allow you to connect to the Ethereum network and interact with your smart contract.
- Connect to the Ethereum Network: Set up a provider to connect to the Ethereum network:
language="language-javascript"const { ethers } = require("ethers");-a1b2c3- const provider = new ethers.providers.Web3Provider(window.ethereum);
- Create a Contract Instance: Use the contract's ABI and address to create an instance:
language="language-javascript"const contract = new ethers.Contract(contractAddress, contractABI, provider);
- Read Data from the Contract: Call functions to read data, such as checking balances:
language="language-javascript"const balance = await contract.balanceOf(userAddress);
- Send Transactions: To transfer tokens, use the contract's transfer function:
language="language-javascript"const tx = await contract.transfer(recipientAddress, amount);-a1b2c3- await tx.wait();
By following these steps, you can effectively interact with your deployed token, enabling users to engage with your smart contract seamlessly. Rapid Innovation supports clients in this interaction phase, ensuring that they can leverage their deployed tokens to achieve their business objectives efficiently. Additionally, utilizing smart contract verification tools like bsc verified contracts and etherscan contract verification can further enhance the trustworthiness of your deployed contracts.
7.1. Using Hardhat console to interact with the token
The Hardhat console is a powerful tool for developers working with Ethereum smart contracts. It allows you to interact with your deployed contracts directly from the command line, making it easier to test and debug your token functionalities.
- Start by installing Hardhat if you haven't already:
language="language-bash"npm install --save-dev hardhat
- Create a new Hardhat project:
language="language-bash"npx hardhat
- Launch the Hardhat console:
language="language-bash"npx hardhat console
- In the console, you can interact with your token contract. First, you need to get the contract instance:
language="language-javascript"const Token = await ethers.getContractFactory("YourToken");-a1b2c3- const token = await Token.attach("YOUR_CONTRACT_ADDRESS");
- Now, you can call functions on your token contract. For example, to check the total supply:
language="language-javascript"const totalSupply = await token.totalSupply();-a1b2c3- console.log(totalSupply.toString());
- You can also check balances, transfer tokens, and approve spending:
language="language-javascript"const balance = await token.balanceOf("YOUR_WALLET_ADDRESS");-a1b2c3- console.log(balance.toString());-a1b2c3--a1b2c3- const tx = await token.transfer("RECIPIENT_ADDRESS", ethers.utils.parseUnits("10", 18));-a1b2c3- await tx.wait();
Using the Hardhat console streamlines the process of testing your token's functionalities, allowing for quick iterations and debugging. This efficiency is crucial for businesses looking to leverage blockchain technology, as it reduces development time and costs, ultimately leading to a greater return on investment (ROI).
7.2. Creating a simple frontend to showcase token functionality
Building a simple frontend can help you visualize and interact with your token. You can use frameworks like React or Vue.js to create a user-friendly interface.
- Set up a new React project:
language="language-bash"npx create-react-app token-frontend-a1b2c3- cd token-frontend
- Install ethers.js to interact with your smart contract:
language="language-bash"npm install ethers
- Create a new component to display token information:
language="language-javascript"import React, { useEffect, useState } from 'react';-a1b2c3- import { ethers } from 'ethers';-a1b2c3- import YourToken from './artifacts/contracts/YourToken.sol/YourToken.json';-a1b2c3--a1b2c3- const TokenInfo = () => {-a1b2c3- const [totalSupply, setTotalSupply] = useState(0);-a1b2c3- const [balance, setBalance] = useState(0);-a1b2c3--a1b2c3- useEffect(() => {-a1b2c3- const fetchData = async () => {-a1b2c3- const provider = new ethers.providers.Web3Provider(window.ethereum);-a1b2c3- const signer = provider.getSigner();-a1b2c3- const tokenContract = new ethers.Contract("YOUR_CONTRACT_ADDRESS", YourToken.abi, signer);-a1b2c3--a1b2c3- const supply = await tokenContract.totalSupply();-a1b2c3- setTotalSupply(supply.toString());-a1b2c3--a1b2c3- const userAddress = await signer.getAddress();-a1b2c3- const userBalance = await tokenContract.balanceOf(userAddress);-a1b2c3- setBalance(userBalance.toString());-a1b2c3- };-a1b2c3--a1b2c3- fetchData();-a1b2c3- }, []);-a1b2c3--a1b2c3- return (-a1b2c3- <div>-a1b2c3- # Token Information-a1b2c3- <p>Total Supply: {totalSupply}</p>-a1b2c3- <p>Your Balance: {balance}</p>-a1b2c3- </div>-a1b2c3- );-a1b2c3- };-a1b2c3--a1b2c3- export default TokenInfo;
- Integrate this component into your main application file (e.g., App.js):
language="language-javascript"import React from 'react';-a1b2c3- import TokenInfo from './TokenInfo';-a1b2c3--a1b2c3- function App() {-a1b2c3- return (-a1b2c3- <div className="App">-a1b2c3- <TokenInfo />-a1b2c3- </div>-a1b2c3- );-a1b2c3- }-a1b2c3--a1b2c3- export default App;
This simple frontend allows users to view the total supply and their token balance, showcasing the token's functionality effectively. By providing a clear and intuitive interface, businesses can enhance user engagement and satisfaction, leading to improved customer retention and increased ROI.
7.3. Implementing token transfers and approvals
Token transfers and approvals are essential functionalities in any ERC20 token. They allow users to send tokens and authorize others to spend tokens on their behalf.
- To implement token transfers, you can add a function in your frontend:
language="language-javascript"const transferTokens = async (recipient, amount) => {-a1b2c3- const provider = new ethers.providers.Web3Provider(window.ethereum);-a1b2c3- const signer = provider.getSigner();-a1b2c3- const tokenContract = new ethers.Contract("YOUR_CONTRACT_ADDRESS", YourToken.abi, signer);-a1b2c3--a1b2c3- const tx = await tokenContract.transfer(recipient, ethers.utils.parseUnits(amount, 18));-a1b2c3- await tx.wait();-a1b2c3- console.log("Transfer successful!");-a1b2c3- };
- For approvals, you can create a similar function:
language="language-javascript"const approveTokens = async (spender, amount) => {-a1b2c3- const provider = new ethers.providers.Web3Provider(window.ethereum);-a1b2c3- const signer = provider.getSigner();-a1b2c3- const tokenContract = new ethers.Contract("YOUR_CONTRACT_ADDRESS", YourToken.abi, signer);-a1b2c3--a1b2c3- const tx = await tokenContract.approve(spender, ethers.utils.parseUnits(amount, 18));-a1b2c3- await tx.wait();-a1b2c3- console.log("Approval successful!");-a1b2c3- };
These functions allow users to transfer tokens and approve others to spend their tokens, enhancing the overall functionality of your token application. By implementing these features, businesses can facilitate seamless transactions and foster trust among users, ultimately driving higher ROI through increased transaction volume and user satisfaction.
8. Preparing for Mainnet Deployment
Deploying a token on the mainnet is a critical step in the lifecycle of a blockchain project. It requires thorough preparation to ensure security, efficiency, and cost-effectiveness.
8.1. Auditing your token contract for security
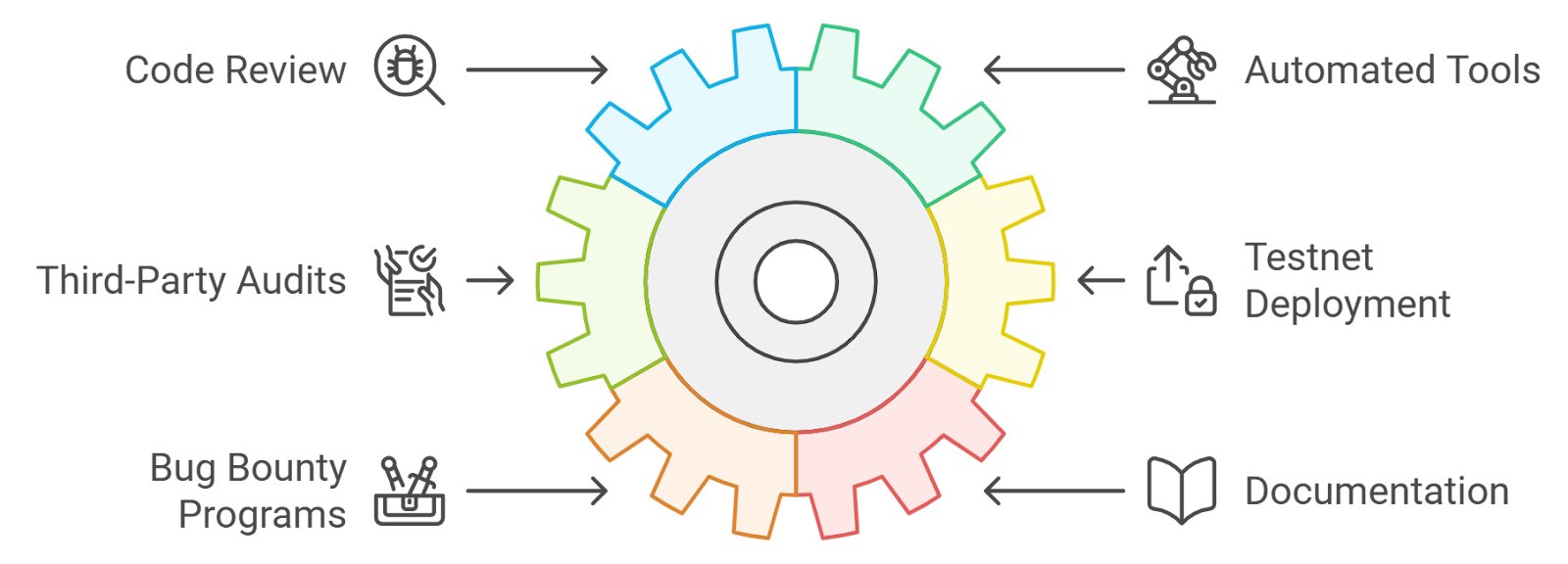
Security is paramount when deploying a token contract. A well-audited contract minimizes vulnerabilities that could be exploited by malicious actors. Here are key steps to ensure your token contract is secure:
- Code Review: Conduct a thorough review of the code to identify any potential vulnerabilities, including common issues such as reentrancy attacks, integer overflows, and improper access control.
- Automated Tools: Utilize automated security tools like MythX, Slither, or Oyente to scan your smart contract for known vulnerabilities. These tools can help identify issues that may not be immediately apparent during manual reviews.
- Third-Party Audits: Engage a reputable third-party auditing firm to conduct a comprehensive audit of your token contract. Firms like ConsenSys Diligence or OpenZeppelin have extensive experience in smart contract security and can provide valuable insights.
- Testnet Deployment: Before going live on the mainnet, deploy your token on a testnet. This allows you to test the contract in a simulated environment, helping to identify any issues that may arise during actual use. You can deploy erc20 token to testnet or deploy erc721 on polygon to ensure functionality.
- Bug Bounty Programs: Consider launching a bug bounty program to incentivize the community to find and report vulnerabilities. This can be an effective way to uncover issues that may have been overlooked.
- Documentation: Maintain clear and comprehensive documentation of your token contract. This not only aids in the auditing process but also helps future developers understand the codebase.
At Rapid Innovation, we specialize in guiding clients through this critical phase, ensuring that your token contract is not only secure but also aligned with best practices in the industry. Our team of experts can assist in conducting thorough audits and implementing robust security measures, ultimately enhancing your project's credibility and potential for success.
8.2. Optimizing gas costs for Arbitrum
When deploying on Arbitrum, optimizing gas costs is essential for ensuring that transactions remain affordable for users. Here are strategies to optimize gas costs:
- Efficient Code: Write efficient smart contract code by avoiding unnecessary computations and storage operations, as these can significantly increase gas costs. This is particularly important when you deploy erc20 token or deploy bep20 token.
- Batch Transactions: Where possible, batch multiple transactions into a single call. This reduces the overall gas fees by minimizing the number of transactions processed.
- Use Libraries: Leverage established libraries like OpenZeppelin for common functionalities. These libraries are optimized for gas efficiency and can save you from reinventing the wheel.
- Gas Limit Settings: Set appropriate gas limits for your transactions. Overestimating can lead to wasted gas, while underestimating can cause transactions to fail.
- Monitor Gas Prices: Keep an eye on current gas prices and deploy during times of lower network congestion. Tools like EthGasStation can help you track gas prices in real-time.
- Layer 2 Solutions: Consider utilizing Layer 2 solutions like Arbitrum to reduce gas costs. These solutions are designed to handle transactions off the main Ethereum chain, significantly lowering fees.
By following these steps, you can ensure that your token contract is secure and optimized for deployment on the Arbitrum network. This preparation is crucial for a successful mainnet launch, providing a solid foundation for your project’s future. At Rapid Innovation, we are committed to helping our clients navigate these complexities, ensuring that your deployment is not only efficient but also maximizes your return on investment. Whether you are looking to deploy erc20 token to mainnet or deploy token on binance smart chain, we have the expertise to assist you.
8.3. Estimating Deployment and Transaction Costs
When deploying a token on the Arbitrum network, it is essential to accurately estimate the token deployment costs and transaction costs. These costs can vary based on several factors, including network congestion, gas prices, and the complexity of your smart contract.
- Gas Fees: The primary cost associated with deploying a token is the gas fees. Gas is the unit that measures the computational effort required to execute operations on the Ethereum network. On Arbitrum, gas fees are generally lower than on the Ethereum mainnet due to its Layer 2 scaling solution. However, they can still fluctuate based on network demand.
- Deployment Costs: The cost to deploy a smart contract can be estimated by calculating the gas required for deployment multiplied by the current gas price. For example, if deploying your token requires 200,000 gas and the gas price is 0.01 ETH, the deployment cost would be:
language="language-plaintext"Deployment Cost = Gas Required * Gas Price-a1b2c3-Deployment Cost = 200,000 * 0.01 = 2,000 ETH
- Transaction Costs: Each transaction involving your token (e.g., transfers, approvals) will also incur gas fees. It is essential to consider these ongoing costs when estimating the overall budget for your token project.
- Tools for Estimation: Utilize tools to monitor current gas prices and estimate costs effectively. For more information on wallet creation and management, you can refer to this step-by-step guide.
9. Deploying Your Token to Arbitrum Mainnet
Deploying your token to the Arbitrum mainnet involves several steps. Arbitrum provides a more efficient and cost-effective environment for token deployment compared to the Ethereum mainnet. Here’s how to proceed:
- Prepare Your Smart Contract: Ensure your token's smart contract is thoroughly tested on a testnet like Arbitrum Rinkeby or Goerli. This step is crucial to avoid costly mistakes during deployment.
- Set Up Your Development Environment: Use tools like Hardhat or Truffle to manage your deployment process. These frameworks simplify the deployment of smart contracts.
- Connect to Arbitrum Mainnet: Configure your wallet (e.g., MetaMask) to connect to the Arbitrum mainnet. Ensure you have the correct RPC URL and network ID.
- Deploy the Contract: Use the following steps to deploy your token:
language="language-plaintext"1. Compile your smart contract using Hardhat or Truffle.-a1b2c3-2. Run the deployment script, specifying the Arbitrum mainnet.-a1b2c3-3. Confirm the transaction in your wallet.-a1b2c3-4. Wait for the transaction to be mined.
- Verify Your Contract: After deployment, verify your contract on Arbiscan to ensure transparency and trustworthiness.
9.1. Acquiring Mainnet ETH for Deployment
To deploy your token on the Arbitrum mainnet, you may need to acquire ETH for gas fees. Here’s how to do it:
- Create a Wallet: If you don’t already have one, create a wallet that supports Ethereum and Arbitrum, such as MetaMask.
- Purchase ETH: You can buy ETH from various exchanges. Ensure you transfer the ETH to your wallet.
- Bridge ETH to Arbitrum: If you have ETH on the Ethereum mainnet, you can use the Arbitrum Bridge to transfer your ETH to the Arbitrum network. Follow these steps:
language="language-plaintext"1. Go to the Arbitrum Bridge website.-a1b2c3-2. Connect your wallet.-a1b2c3-3. Select the amount of ETH to bridge.-a1b2c3-4. Confirm the transaction and wait for it to complete.
- Check Your Balance: After bridging, check your wallet to ensure the ETH is available on the Arbitrum network.
By following these steps, you can effectively estimate token deployment costs, deploy your token, and acquire the necessary ETH for a successful deployment on the Arbitrum mainnet. Rapid Innovation is here to assist you throughout this process, ensuring that your deployment is efficient and aligned with your business goals, ultimately leading to greater ROI.
9.2. Modifying the deployment script for mainnet
When preparing to deploy your application on the mainnet, it is crucial to modify your deployment script to ensure it aligns with the mainnet's requirements. This involves several key adjustments:
- Update Network Configuration: Ensure that the network settings in your script point to the mainnet. This typically includes changing the network ID and RPC URLs.
- Adjust Gas Settings: Mainnet transactions often require higher gas limits and gas prices compared to testnets. Update these parameters in your script to avoid transaction failures.
- Environment Variables: Set environment variables that are specific to the mainnet. This may include private keys, contract addresses, and other sensitive information.
- Contract Addresses: If your deployment script references any existing contracts, ensure that you are using the correct mainnet addresses.
- Testing: Before finalizing the script, conduct thorough testing on a testnet to ensure that all modifications work as intended.
Example of a modified deployment script snippet:
language="language-javascript"const Web3 = require('web3');-a1b2c3--a1b2c3-const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'));-a1b2c3--a1b2c3-const contract = new web3.eth.Contract(ABI, '0xYourMainnetContractAddress');
9.3. Executing the mainnet deployment
Once your deployment script is modified and tested, you can proceed to execute the mainnet deployment. This step is critical and should be approached with caution.
- Backup Important Data: Before executing the deployment, ensure that you have backups of all important data, including private keys and contract addresses.
- Connect to Mainnet: Use a reliable node provider like Infura or Alchemy to connect to the Ethereum mainnet.
- Run the Deployment Script: Execute the modified deployment script. Monitor the console for any errors or warnings during the process.
- Verify Deployment: After execution, verify that your contracts are deployed correctly. You can use tools like Etherscan to check the status of your transactions.
- Monitor Transactions: Keep an eye on the transaction status to ensure that everything is functioning as expected.
Example command to execute the deployment script:
language="language-bash"node deploy.js
10. Post-Deployment Steps and Best Practices
After successfully deploying your application on the mainnet, it is essential to follow up with post-deployment steps and best practices to ensure the longevity and security of your project.
- Audit Smart Contracts: Conduct a thorough audit of your smart contracts to identify any vulnerabilities. Consider hiring third-party auditors for an unbiased review.
- Monitor Performance: Use monitoring tools to keep track of your application’s performance and transaction metrics. This helps in identifying any issues early on.
- Implement Security Measures: Ensure that you have implemented security best practices, such as using multi-signature wallets for fund management and regularly updating your software.
- Community Engagement: Engage with your community for feedback and support. This can help in identifying issues and improving your application.
- Documentation: Maintain comprehensive documentation for your deployment process and smart contracts. This is crucial for future updates and for onboarding new team members.
- Regular Updates: Keep your application updated with the latest security patches and improvements. Regular updates help in maintaining the integrity of your project.
By following these steps, you can ensure a successful mainnet deployment and maintain the health of your application in the long run.
At Rapid Innovation, we specialize in guiding clients through these critical deployment processes, ensuring that your blockchain applications are not only effectively launched but also optimized for performance and security. Our expertise in AI and blockchain technology allows us to provide tailored solutions that enhance your operational efficiency and maximize your return on investment. Whether you are looking to streamline your deployment process or enhance your application's capabilities, our team is here to support you every step of the way.
10.1. Renouncing ownership or implementing governance
Renouncing ownership of a token or project, such as a rari governance token or a silo governance token, is a significant decision that can enhance trust within the community. By relinquishing control, developers signal their commitment to decentralization. However, this step should be carefully considered, as it can limit the ability to make future changes.
- Benefits of Renouncing Ownership:
- Increases trust among users and investors.
- Reduces the risk of centralized control and manipulation.
- Encourages community-driven development.
- Implementing Governance:
- Instead of renouncing ownership, consider implementing a governance model. This allows token holders to vote on key decisions, such as protocol upgrades or fund allocation. For example, governance token holders like those of the ogv token or mstable governance token can participate in these decisions.
- Steps to Implement Governance:
- Define governance parameters (voting rights, quorum requirements).
- Develop a governance token if necessary, such as the agov token or apy governance token.
- Use platforms like Snapshot for off-chain voting or on-chain solutions like Aragon.
10.2. Adding liquidity to decentralized exchanges on Arbitrum
Adding liquidity to decentralized exchanges (DEXs) on Arbitrum is crucial for ensuring that users can trade your token efficiently. Arbitrum, a layer-2 scaling solution for Ethereum, offers lower fees and faster transactions, making it an attractive option for liquidity provision.
- Benefits of Adding Liquidity:
- Enhances trading volume and price stability.
- Attracts more users to your token, including those interested in the dydx governance or axs governance token.
- Provides liquidity providers with potential rewards.
- Steps to Add Liquidity:
- Choose a DEX on Arbitrum (e.g., Uniswap, SushiSwap).
- Connect your wallet (MetaMask, WalletConnect).
- Select the token pair (your token and ETH or USDC).
- Determine the amount of each token to provide.
- Approve the token for trading.
- Confirm the liquidity provision transaction.
- Considerations:
- Monitor impermanent loss, which can affect your returns.
- Consider incentivizing liquidity providers with rewards or token emissions, similar to those offered by bancor governance token or curio governance token.
10.3. Marketing and community building for your token
Effective marketing and community building are essential for the success of your token. A strong community can drive adoption, provide feedback, and create a sense of ownership among users.
- Strategies for Marketing:
- Utilize social media platforms (Twitter, Telegram, Discord) to engage with potential users.
- Create informative content (blogs, videos) to educate the community about your token's use case, including the governance token meaning and governance token list.
- Collaborate with influencers in the crypto space to reach a broader audience.
- Community Building Techniques:
- Host AMAs (Ask Me Anything) to address community questions and concerns.
- Organize events or contests to encourage participation and engagement.
- Establish a feedback loop to gather insights from the community and implement suggestions.
- Tools for Community Engagement:
- Use platforms like Discord for real-time communication.
- Leverage Telegram for announcements and updates.
- Create a dedicated subreddit for discussions and community-driven content.
By focusing on these areas, you can create a robust ecosystem around your token, ensuring its long-term success and sustainability. At Rapid Innovation, we leverage our expertise in AI and Blockchain to guide clients through these processes, ensuring they achieve their business goals efficiently and effectively. Our tailored solutions can help you maximize ROI by implementing effective governance models, enhancing liquidity, and building a vibrant community around your token, including those related to ogv token price and bcug token.
11. Troubleshooting Common Issues
11.1. Dealing with failed transactions on Arbitrum

Failed transactions on Arbitrum can be frustrating, but understanding the common causes can help you troubleshoot effectively. Here are some typical reasons for transaction failures and how to address them:
- Insufficient Gas Fees: Transactions may fail if the gas limit is set too low. Always ensure that you allocate enough gas for your transaction.
- Network Congestion: High traffic on the Arbitrum network can lead to delays or failures. Check the network status and consider resubmitting your transaction during off-peak hours.
- Smart Contract Issues: If the transaction involves a smart contract, ensure that the contract is functioning correctly. Review the contract code for any bugs or issues.
- Nonce Issues: Each transaction has a unique nonce. If you attempt to send multiple transactions with the same nonce, the subsequent transactions will fail. Make sure to increment the nonce for each new transaction.
- Token Approval: If you are interacting with a token contract, ensure that you have approved the contract to spend your tokens. Without proper approval, the transaction will fail.
To troubleshoot failed transactions, follow these steps:
- Check the transaction status on a block explorer.
- Review the error message provided by the wallet or interface you are using.
- Adjust the gas limit and resubmit the transaction.
- If using a smart contract, debug the contract code to identify any issues.
- Ensure that the nonce is correct and incremented for each transaction.
If you encounter troubleshooting failed transactions, it is essential to systematically address each potential issue to identify the root cause.
11.2. Resolving contract verification errors
Contract verification errors can occur when deploying or interacting with smart contracts on Arbitrum. These errors can prevent users from verifying their contracts on block explorers. Here are some common issues and solutions:
- Incorrect Compiler Version: Ensure that you are using the correct compiler version that matches the one used during contract deployment. Mismatched versions can lead to verification failures.
- Missing Constructor Arguments: If your contract has constructor parameters, you must provide the correct arguments during verification. Double-check the values and types of the arguments.
- Source Code Mismatch: The source code you provide for verification must match the deployed bytecode. If there are any discrepancies, the verification will fail. Always ensure that the code is identical.
- Optimization Settings: If you used optimization during compilation, make sure to enable the same settings during verification. This can affect the bytecode and lead to mismatches.
To resolve contract verification errors, follow these steps:
- Confirm the compiler version used for deployment and adjust your settings accordingly.
- Gather the correct constructor arguments and ensure they are formatted properly.
- Compare the deployed bytecode with your source code to ensure they match.
- Check the optimization settings and ensure they are consistent with your deployment.
By addressing these common issues, you can effectively troubleshoot failed transactions and contract verification errors on Arbitrum, ensuring a smoother experience in your blockchain interactions. At Rapid Innovation, we leverage our expertise in AI and Blockchain to provide tailored solutions that help clients navigate these challenges, ultimately enhancing their operational efficiency and maximizing ROI.
11.3. Handling Cross-Chain Bridging Issues
Cross-chain bridging is a critical aspect of blockchain interoperability, allowing assets and data to move seamlessly between different blockchain networks. However, several challenges can arise during this process. Addressing these issues is essential for ensuring a smooth user experience and maintaining the integrity of transactions.
- Security Risks: Cross-chain bridges can be vulnerable to hacks and exploits. To mitigate these risks:
- Utilize multi-signature wallets for transaction approvals.
- Implement robust auditing processes for smart contracts.
- Regularly update and patch vulnerabilities in the bridge protocol.
- Liquidity Challenges: Insufficient liquidity can hinder the effectiveness of cross-chain transactions. To enhance liquidity:
- Encourage liquidity providers by offering incentives such as yield farming.
- Collaborate with decentralized exchanges (DEXs) to facilitate better liquidity pools.
- Monitor liquidity levels and adjust incentives accordingly.
- User Experience: Complicated processes can deter users from utilizing cross-chain bridges. To improve user experience:
- Simplify the interface for initiating cross-chain transactions.
- Provide clear instructions and support for users unfamiliar with the process.
- Implement automated systems that minimize the number of steps required for bridging.
- Interoperability Standards: Different blockchains may have varying standards, leading to compatibility issues. To address this:
- Adopt widely accepted standards like the Inter-Blockchain Communication (IBC) protocol.
- Engage in community discussions to establish common protocols for cross-chain interactions.
- Develop middleware solutions that can translate between different blockchain protocols.
12. Advanced Topics and Future Developments
As the blockchain ecosystem evolves, advanced topics and future developments in cross-chain technology are becoming increasingly relevant. These advancements can significantly enhance the functionality and usability of blockchain networks.
- Layer 2 Solutions: Layer 2 solutions, such as rollups and state channels, can improve transaction speeds and reduce costs. Future developments may focus on:
- Integrating Layer 2 solutions with cross-chain bridges to enhance scalability.
- Exploring hybrid models that combine Layer 1 and Layer 2 functionalities.
- Decentralized Autonomous Organizations (DAOs): DAOs can play a pivotal role in managing cross-chain protocols. Future developments may include:
- Establishing DAOs to govern cross-chain bridge operations and decision-making.
- Utilizing smart contracts to automate governance processes and enhance transparency.
- Interoperable DeFi Ecosystems: The future of decentralized finance (DeFi) lies in creating interoperable ecosystems. Key areas of focus may include:
- Developing cross-chain lending and borrowing platforms that allow users to leverage assets across multiple chains, including avalanche cross chain and axelar cross chain.
- Creating cross-chain liquidity pools that enable users to trade assets seamlessly, such as those on polygon cross chain and binance cross chain.
12.1. Implementing Cross-Chain Functionality for Your Token
Implementing cross-chain functionality for your token can significantly enhance its utility and market reach. Here are steps to achieve this:
- Choose the Right Protocol: Select a cross-chain protocol that aligns with your token's goals. Popular options include:
- Polkadot
- Cosmos
- Avalanche, which is known for its cross-chain capabilities.
- Develop Smart Contracts: Create smart contracts that facilitate cross-chain transactions. Key considerations include:
- Ensuring compatibility with the chosen protocol.
- Implementing security measures to protect against vulnerabilities.
- Test the Implementation: Conduct thorough testing to ensure the cross-chain functionality works as intended. Steps include:
- Running test transactions on testnets.
- Engaging in bug bounty programs to identify potential issues.
- Launch and Monitor: Once testing is complete, launch the cross-chain functionality. Ongoing monitoring is crucial:
- Track transaction performance and user feedback.
- Regularly update the system to address any emerging issues.
By addressing these aspects, you can effectively implement cross-chain functionality for your token, enhancing its value and usability in the growing blockchain ecosystem. At Rapid Innovation, we specialize in providing tailored solutions to help you navigate these complexities, ensuring that your cross-chain initiatives yield maximum ROI and align with your business objectives. This includes leveraging technologies like harmony cross chain, synapse cross chain, and wormhole cross chain to enhance your project's interoperability.
12.2. Exploring Arbitrum Nova for Gaming and Social Tokens

Arbitrum Nova is a layer-2 scaling solution designed to enhance the Ethereum blockchain's capabilities, particularly for gaming and social tokens. Its unique architecture allows for lower transaction fees and faster processing times, making it an attractive option for developers and users alike.
- Low Transaction Costs: Arbitrum Nova significantly reduces gas fees, which is crucial for gaming applications where microtransactions are common. This affordability encourages more users to participate in the ecosystem, ultimately driving higher engagement and revenue potential.
- High Throughput: The platform can handle a large number of transactions per second, which is essential for gaming environments that require real-time interactions. This scalability ensures that games can operate smoothly without lag, enhancing user experience and retention.
- Developer-Friendly Environment: Arbitrum Nova supports Ethereum's existing tools and infrastructure, making it easier for developers to migrate their projects. This compatibility allows for a seamless transition and encourages innovation in the gaming and social token sectors, leading to faster time-to-market and reduced development costs.
- Community Engagement: Social tokens can thrive on Arbitrum Nova due to its ability to facilitate community-driven projects. Creators can launch tokens that represent their brand or community, fostering engagement and loyalty among users, which can translate into increased monetization opportunities.
- Interoperability: The platform's design allows for easy integration with other blockchain networks, enhancing the potential for cross-platform gaming experiences and social interactions. This interoperability can open new revenue streams and user bases for developers.
To explore Arbitrum Nova for gaming and social tokens, developers can follow these steps:
- Research existing projects on Arbitrum Nova to understand the ecosystem.
- Set up a development environment using tools like Hardhat or Truffle.
- Create a smart contract for a gaming or social token using Solidity.
- Deploy the contract on Arbitrum Nova using a wallet like MetaMask.
- Test the application thoroughly to ensure functionality and user experience.
12.3. Staying Updated with Arbitrum Ecosystem Developments
Keeping abreast of the latest developments in the Arbitrum ecosystem is vital for developers, investors, and users. The rapidly evolving nature of blockchain technology means that new features, updates, and projects can emerge frequently.
- Official Channels: Follow Arbitrum's official blog and social media accounts for announcements and updates. These platforms often provide insights into upcoming features and community events.
- Community Forums: Engage with the Arbitrum community on platforms like Discord and Reddit. These forums are excellent for networking, sharing knowledge, and staying informed about user experiences and project developments.
- News Aggregators: Utilize blockchain news websites and aggregators to get the latest information on Arbitrum and its ecosystem. Websites like CoinDesk and The Block often cover significant updates and trends.
- Developer Resources: Regularly check the Arbitrum documentation and GitHub repositories for technical updates and new tools. This information is crucial for developers looking to leverage the latest features in their projects.
- Webinars and Conferences: Participate in webinars and blockchain conferences that focus on Arbitrum and layer-2 solutions. These events provide valuable insights from industry experts and opportunities for networking.
To stay updated, consider these steps:
- Subscribe to newsletters from Arbitrum and related blockchain news sites.
- Join relevant Discord channels and Telegram groups.
- Set up Google Alerts for "Arbitrum" to receive notifications about new articles and developments.
13. Conclusion and Next Steps
In conclusion, exploring Arbitrum Nova for gaming and social tokens presents a wealth of opportunities for developers and users alike. By staying informed about the latest developments in the Arbitrum ecosystem, stakeholders can effectively navigate this dynamic landscape. Engaging with the community and leveraging the platform's capabilities will be essential for maximizing the potential of gaming and social tokens on Arbitrum Nova. At Rapid Innovation, we are committed to guiding our clients through this evolving landscape, ensuring they achieve their business goals efficiently and effectively while maximizing their return on investment. For more information on blockchain technology, you can read about Polygon Blockchain.
13.1. Recap of the token deployment process on Arbitrum
Deploying a token on Arbitrum involves several key steps that leverage the Ethereum-compatible layer-2 solution. The process is designed to be efficient and cost-effective, allowing developers to create and manage tokens with ease.
- Set Up Development Environment:
- Install Node.js and npm.
- Use Truffle or Hardhat for smart contract development.
- Create Smart Contract:
- Write the ERC-20 or ERC-721 token contract in Solidity.
- Ensure to include essential functions like
transfer
,approve
, andmint
.
- Configure Arbitrum Network:
- Add Arbitrum to your wallet (e.g., MetaMask).
- Use the Arbitrum RPC URL for network configuration.
- Deploy the Contract:
- Use Truffle or Hardhat to deploy the contract to the Arbitrum network.
- Verify the deployment on Arbitrum's block explorer.
- Interact with the Token:
- Use
web3.js
orethers.js
to interact with your deployed token. - Test functionalities like transfers and balance checks.
- Use
This streamlined process allows developers to take advantage of Arbitrum's scalability and lower transaction fees compared to Ethereum's mainnet. At Rapid Innovation, we specialize in guiding clients through this deployment process, ensuring that they can efficiently launch their token projects while maximizing their return on investment (ROI). If you're looking for expert assistance, consider our blockchain app development services to help you navigate this process effectively.
13.2. Resources for further learning and community engagement
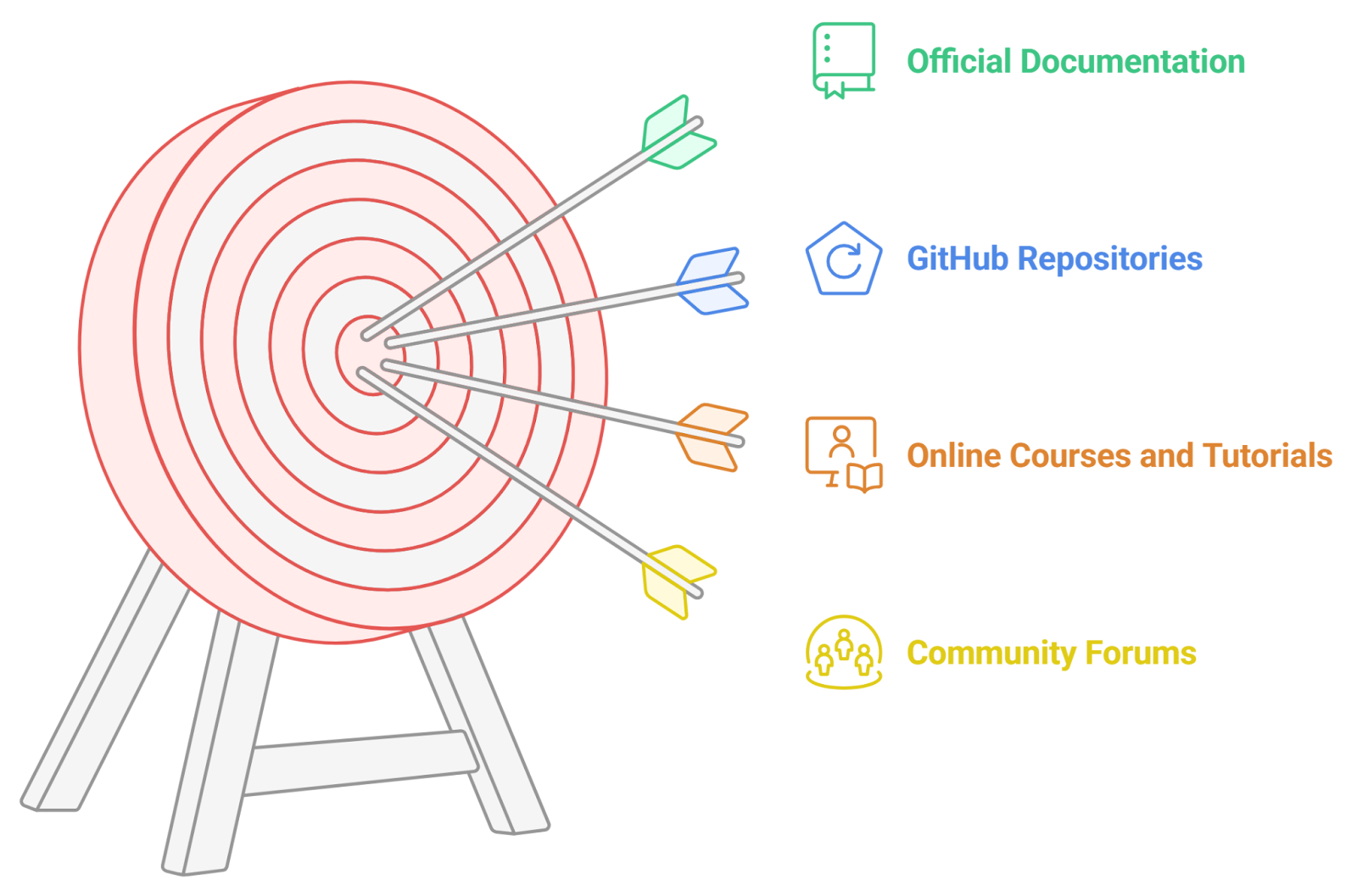
To deepen your understanding of Arbitrum and engage with the community, several resources are available:
- Official Documentation:
- The Arbitrum Developer Documentation provides comprehensive guides on deploying and interacting with smart contracts.
- Community Forums:
- Join the Arbitrum Discord to connect with other developers and enthusiasts.
- Participate in discussions on platforms like Reddit and Stack Overflow.
- Online Courses and Tutorials:
- Platforms like Coursera and Udemy offer courses on Ethereum and layer-2 solutions.
- YouTube channels dedicated to blockchain development often feature tutorials on Arbitrum.
- GitHub Repositories:
- Explore open-source projects on GitHub that utilize Arbitrum to learn from real-world implementations.
Engaging with these resources will enhance your knowledge and keep you updated on the latest developments in the Arbitrum ecosystem. Rapid Innovation also provides tailored training and consulting services to help clients navigate these resources effectively.
13.3. Exciting possibilities for your Arbitrum-based token project
The deployment of a token on Arbitrum opens up a myriad of exciting possibilities for developers and entrepreneurs:
- Lower Transaction Costs:
- Benefit from significantly reduced gas fees, making microtransactions feasible.
- Scalability:
- Handle a larger volume of transactions without congestion, ideal for applications requiring high throughput.
- Interoperability:
- Leverage Ethereum's ecosystem while enjoying the advantages of layer-2 solutions, allowing for seamless integration with existing dApps.
- Innovative Use Cases:
- Explore unique applications such as decentralized finance (DeFi), non-fungible tokens (NFTs), and gaming, all enhanced by Arbitrum's capabilities.
- Community Building:
- Foster a community around your token project, utilizing social media and forums to engage users and gather feedback.
By harnessing these possibilities, your Arbitrum-based token project can thrive in a competitive landscape, attracting users and investors alike. Rapid Innovation is here to support you in realizing these opportunities, ensuring that your project not only meets but exceeds your business goals.