Table Of Contents
Category
Security
Artificial Intelligence
AIML
IoT
1. Introduction to Solana Smart Contract Testing and Debugging
At Rapid Innovation, we understand that Solana is a high-performance blockchain platform designed for decentralized applications and crypto projects. Smart contracts on Solana, known as programs, require rigorous testing and debugging to ensure they function correctly and securely. This guide will explore the essential aspects of testing and debugging Solana smart contracts, showcasing how our expertise can help you achieve your development goals efficiently and effectively.
1.1. Why Testing and Debugging are Crucial for Solana Development
Testing and debugging are vital components of the development lifecycle for several reasons:
- Security: Smart contracts are immutable once deployed. Any vulnerabilities can lead to significant financial losses. According to a report, over $1.3 billion was lost to smart contract exploits in 2021 alone. Ensuring robust testing can mitigate these risks, and our team at Rapid Innovation specializes in implementing comprehensive security measures to protect your investments.
- Performance: Solana is known for its high throughput and low latency. Testing helps identify performance bottlenecks, ensuring that the smart contracts can handle the expected load without degradation. Our development strategies are tailored to optimize performance, ensuring you achieve greater ROI.
- User Trust: Well-tested and debugged contracts foster user confidence. If users know that a contract has undergone thorough testing, they are more likely to interact with it. By partnering with us, you can enhance user trust and engagement, leading to increased adoption of your applications.
- Cost Efficiency: Debugging after deployment can be costly and time-consuming. Identifying issues during the development phase saves resources and time. Our proactive approach to testing ensures that you minimize costs and maximize efficiency.
- Compliance: As regulations around blockchain technology evolve, ensuring that smart contracts comply with legal standards is essential. Testing can help identify compliance issues early, and our consulting services can guide you through the regulatory landscape.
Testing Strategies for Solana Smart Contracts
- Unit Testing:
- Write tests for individual functions to ensure they behave as expected.
- Use frameworks like Mocha or Jest for JavaScript-based testing.
- Integration Testing:
- Test how different components of the smart contract interact with each other.
- Ensure that the contract works well with external systems and APIs.
- End-to-End Testing:
- Simulate real-world scenarios to test the entire application flow.
- Use tools like Cypress or Selenium for comprehensive testing.
Debugging Techniques for Solana Smart Contracts
- Logging:
- Implement logging within the smart contract to track the flow of execution and variable states.
- Use the msg! macro to log messages in Solana programs.
- Error Handling:
- Use proper error handling to catch and manage exceptions gracefully.
- Implement custom error types to provide more context on failures.
- Local Development Environment:
- Set up a local Solana cluster using solana-test-validator for testing.
- This allows for rapid iteration and debugging without incurring transaction costs.
Tools for Testing and Debugging
- Anchor Framework:
- A framework for Solana smart contracts that simplifies development and testing.
- Provides built-in testing utilities and a structured way to write smart contracts.
- Solana CLI:
- Command-line interface for interacting with the Solana blockchain.
- Useful for deploying contracts and running tests.
- Solana Explorer:
- A web-based tool to view transactions and contract states on the Solana blockchain.
- Helps in debugging by providing insights into transaction history and contract interactions.
Best Practices for Testing and Debugging
- Automate Tests:
- Use Continuous Integration (CI) tools to automate testing processes.
- Ensure that tests run on every code change to catch issues early.
- Write Clear Documentation:
- Document the purpose and functionality of each smart contract.
- Include instructions on how to run tests and debug issues.
- Conduct Code Reviews:
- Regularly review code with peers to identify potential issues.
- Encourage collaborative debugging sessions to leverage collective knowledge.
By mastering testing and debugging techniques, developers can create secure, efficient, and reliable smart contracts on the Solana blockchain. At Rapid Innovation, we are committed to helping you navigate this complex landscape, ultimately contributing to the ecosystem's growth and user trust while ensuring you achieve greater ROI through our tailored solutions. Partner with us to unlock the full potential of your blockchain projects. For more insights, check out the Ultimate Guide to Testing and Debugging Rust Code in 2024: Boost Your Skills Now.
1.2. Overview of Solana's Programming Model
Solana's programming model is designed to facilitate high-performance decentralized applications (dApps) and smart contracts. It leverages a unique architecture that allows for scalability and speed, making it a popular choice among developers.
- Account-based Model: Solana uses an account-based model where each account can hold data and execute instructions. This model simplifies the interaction between smart contracts and accounts.
- Parallel Processing: Solana employs a mechanism called Sealevel, which allows for parallel transaction processing. This means multiple transactions can be executed simultaneously, significantly increasing throughput.
- Rust and C: Smart contracts on Solana, known as programs, are primarily written in Rust and C. These languages provide performance and safety, making them suitable for blockchain development.
- Transaction Fees: Solana's fee structure is designed to be low, allowing developers to deploy and interact with smart contracts without incurring high costs. This encourages experimentation and innovation.
- Program Derived Addresses (PDAs): PDAs are unique addresses that can be generated from a program's public key and a seed. They allow for more complex interactions and state management within smart contracts.
1.3. Common Challenges in Solana Smart Contract Development
While Solana offers a robust platform for smart contract development, developers often face several challenges:
- Learning Curve: The use of Rust and C can be daunting for developers who are more familiar with languages like JavaScript or Python. Understanding the nuances of these languages is crucial for effective development.
- Debugging: Debugging smart contracts can be complex due to the asynchronous nature of blockchain transactions. Developers may find it challenging to trace errors and understand transaction failures.
- State Management: Managing state across multiple accounts can be tricky. Developers need to ensure that the state is consistent and correctly updated, which requires careful planning and implementation.
- Limited Documentation: Although Solana has been growing rapidly, some developers find the documentation lacking in certain areas, making it difficult to find solutions to specific problems.
- Ecosystem Maturity: As a relatively new platform, the Solana ecosystem is still maturing. Developers may encounter issues related to libraries, tools, and community support that are not as robust as those found in more established ecosystems.
2. Setting Up Your Solana Development Environment
Setting up a Solana development environment is essential for building and deploying smart contracts. Here are the steps to get started:
- Install Rust:
- Download and install Rust using rustup, which manages Rust versions and associated tools.
- Run the command:
language="language-bash"curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Install Solana CLI:
- Use the following command to install the Solana Command Line Interface (CLI):
language="language-bash"sh -c "$(curl -sSfL https://release.solana.com/v1.10.32/install)"
- Set Up Your Wallet:
- Create a new wallet using the Solana CLI:
language="language-bash"solana-keygen new --outfile ~/my-wallet.json
- Configure the CLI:
- Set the CLI to use the devnet for testing:
language="language-bash"solana config set --url https://api.devnet.solana.com
- Install Anchor:
- Anchor is a framework for Solana smart contract development. Install it using Cargo:
language="language-bash"cargo install --git https://github.com/project-serum/anchor anchor-cli --locked
- Create a New Project:
- Use Anchor to create a new project:
language="language-bash"anchor init my_project
- Build and Deploy:
- Navigate to your project directory and build the project:
language="language-bash"cd my_project-a1b2c3-anchor build
- Deploy the smart contract to the devnet:
language="language-bash"anchor deploy
By following these steps, developers can set up a functional Solana development environment and begin building their decentralized applications.
At Rapid Innovation, we understand the complexities involved in navigating the Solana programming model and the challenges that developers face. Our team of experts is equipped to guide you through the intricacies of Solana development, ensuring that you can leverage its full potential to achieve your business goals efficiently and effectively.
By partnering with us, you can expect:
- Expert Guidance: Our experienced consultants will help you overcome the learning curve associated with Rust and C, ensuring that your team is well-equipped to develop high-performance dApps. For more information, check out our Ultimate Solana Development Guide 2024: Tools, Wallets, Deployment & Best Practices.
- Enhanced Debugging Support: We provide robust debugging solutions that simplify the process of identifying and resolving issues in your smart contracts.
- State Management Solutions: Our team will assist you in implementing effective state management strategies, ensuring consistency and reliability across your applications.
- Comprehensive Documentation: We offer tailored documentation and resources to help your developers navigate the Solana ecosystem with ease.
- Access to a Mature Ecosystem: With our extensive network and resources, you will benefit from a more mature development environment, reducing the risks associated with using a newer platform.
By choosing Rapid Innovation, you are not just investing in development services; you are partnering with a firm dedicated to maximizing your return on investment and driving your success in the blockchain space. Let us help you turn your innovative ideas into reality. For more details on our services, visit our Solana Blockchain Development Company | Rapid Innovation.
2.1. Installing Solana CLI Tools
To interact with the Solana blockchain, it is essential to install the Solana Command Line Interface (CLI) tools. These tools empower you to create wallets, deploy programs, and manage your Solana accounts efficiently.
- Ensure you have the latest version of Node.js installed on your machine.
- Open your terminal and run the following command to install the Solana CLI:
language="language-bash"sh -c "$(curl -sSfL https://release.solana.com/v1.10.32/install)"
- After installation, add the Solana CLI to your system's PATH by adding the following line to your shell configuration file (e.g.,
.bashrc
,.zshrc
):
language="language-bash"export PATH="/home/your_username/.local/share/solana/install/active_release/bin:$PATH"
- Reload your shell configuration:
language="language-bash"source ~/.bashrc
- Verify the installation by checking the version:
language="language-bash"solana --version
This should return the version of the Solana CLI you installed.
2.2. Configuring Your Development Wallet
Once the Solana CLI is installed, the next step is to configure your development wallet. This wallet will be used to send and receive SOL (the native token of Solana) and interact with the blockchain seamlessly.
- Create a new wallet using the following command:
language="language-bash"solana-keygen new
- Follow the prompts to save your seed phrase securely. This phrase is crucial for recovering your wallet.
- Set your wallet as the default keypair:
language="language-bash"solana config set --keypair ~/.config/solana/id.json
- Check your wallet balance (it should be zero initially):
language="language-bash"solana balance
- If you need SOL for testing, you can request some from the Solana devnet faucet:
language="language-bash"solana airdrop 2
This command will send 2 SOL to your wallet on the devnet.
2.3. Setting Up a Local Solana Cluster for Testing
Setting up a local Solana cluster can be beneficial for testing your applications without incurring costs on the mainnet or devnet. This allows you to simulate the Solana environment on your local machine effectively.
- Install the Solana test validator:
language="language-bash"cargo install solana-cli
- Start the local test validator:
language="language-bash"solana-test-validator
- In a new terminal window, set your CLI to connect to the local cluster:
language="language-bash"solana config set --url http://localhost:8899
- Create a new wallet for testing:
language="language-bash"solana-keygen new --no-passphrase
- Set the new wallet as the default keypair:
language="language-bash"solana config set --keypair ~/.config/solana/id.json
- Request some SOL from the local faucet:
language="language-bash"solana airdrop 10
- Verify your balance:
language="language-bash"solana balance
This setup allows you to develop and test your applications in a controlled environment, ensuring that you can innovate rapidly and efficiently.
3. Writing Testable Solana Smart Contracts
3.1. Best Practices for Structuring Your Solana Programs
At Rapid Innovation, we understand that developing smart contracts on the Solana blockchain requires a strategic approach to structuring your programs. Effective structuring is crucial for maintainability and testability, which ultimately leads to greater efficiency and return on investment (ROI). Here are some best practices we recommend:
- Use Clear Naming Conventions:
- Choose descriptive names for your functions and variables to enhance readability.
- Follow a consistent naming pattern to make it easier for others to understand your code.
- Organize Code into Modules:
- Break your code into logical modules that encapsulate specific functionalities.
- This separation allows for easier testing and debugging, reducing the time and cost associated with development.
- Implement Error Handling:
- Use proper error handling mechanisms to manage unexpected situations gracefully.
- This can include using Result types to return errors instead of panicking, which helps maintain system stability.
- Write Unit Tests:
- Create unit tests for each module to ensure that individual components work as expected.
- Utilize the Solana testing framework to simulate transactions and validate outcomes, ensuring reliability and performance.
- Document Your Code:
- Include comments and documentation to explain complex logic and decisions.
- This practice aids future developers in understanding your codebase, facilitating smoother transitions and collaborations.
- Follow the Solana Program Library (SPL) Standards:
- Adhere to the standards set by the SPL to ensure compatibility and interoperability with other Solana programs.
- This can also help in leveraging existing libraries and tools, maximizing your development efforts.
3.2. Implementing Modular and Reusable Code

Creating modular and reusable code is essential for efficient development and testing of Solana smart contracts. At Rapid Innovation, we employ several strategies to achieve this, ensuring our clients benefit from streamlined processes and enhanced ROI:
- Define Clear Interfaces:
- Establish clear interfaces for your modules to define how they interact with each other.
- This promotes loose coupling and makes it easier to swap out implementations, allowing for greater flexibility.
- Use Generic Types:
- Implement generic types in your functions to allow for flexibility and reusability.
- This can help reduce code duplication and improve maintainability, ultimately saving time and resources.
- Create Utility Functions:
- Develop utility functions that can be reused across different modules.
- This minimizes redundancy and centralizes common logic, enhancing overall efficiency.
- Leverage Inheritance and Composition:
- Use inheritance to create base classes that encapsulate shared functionality.
- Alternatively, use composition to build complex behaviors from simpler, reusable components, fostering innovation.
- Test Each Module Independently:
- Write tests for each module separately to ensure they function correctly in isolation.
- This approach simplifies debugging and enhances the reliability of your code, leading to fewer issues in production.
- Version Control for Modules:
- Use version control systems to manage changes in your modules.
- This allows you to track changes and revert to previous versions if necessary, ensuring stability and confidence in your development process.
By following these best practices and strategies, you can create testable, maintainable, and reusable smart contracts on the Solana blockchain. Partnering with Rapid Innovation not only improves the quality of your code but also enhances collaboration and reduces the time required for future development, ultimately driving greater ROI for your projects.
3.3. Handling State and Account Management for Easy Testing
In Solana, managing state and accounts is crucial for effective testing of smart contracts. Proper handling allows developers to simulate various scenarios and ensure that their contracts behave as expected. Here are some key strategies:
- Use Mock Accounts: Create mock accounts to simulate different states. This allows you to test how your smart contract interacts with various account states without affecting the mainnet.
- State Initialization: Ensure that your smart contract has a clear state initialization process. This can be done by defining a setup function that initializes the necessary accounts and state variables before running tests.
- Transaction Simulation: Utilize Solana's transaction simulation features to test how your contract will behave under different conditions. This helps in identifying potential issues before deploying to the mainnet.
- Account Ownership: Manage account ownership carefully. Ensure that the accounts used in tests are owned by the expected program to avoid permission issues during execution.
- State Resetting: Implement a mechanism to reset the state between tests. This ensures that each test runs in a clean environment, preventing state leakage from previous tests.
4. Unit Testing Solana Smart Contracts
Unit testing is essential for ensuring the reliability and correctness of Solana smart contracts. It allows developers to verify that individual components of their contracts function as intended. Here are some best practices for unit testing:
- Test Frameworks: Use testing frameworks like Mocha or Jest, which are compatible with Solana's development environment. These frameworks provide a structured way to write and run tests.
- Isolate Tests: Each test should be independent. Avoid dependencies between tests to ensure that the failure of one test does not affect others.
- Assertions: Use assertions to validate the expected outcomes of your tests. This helps in identifying discrepancies between expected and actual results.
- Edge Cases: Test edge cases and unexpected inputs. This ensures that your smart contract can handle a variety of scenarios without failing.
- Gas Usage: Monitor gas usage during tests. This helps in optimizing the contract and ensuring that it remains efficient.
4.1. Introduction to Solana's Testing Framework
Solana provides a robust testing framework that simplifies the process of writing and executing tests for smart contracts. Understanding this framework is essential for effective unit testing. Key features include:
- Built-in Support: Solana's testing framework comes with built-in support for simulating transactions and accounts, making it easier to test smart contracts in a controlled environment.
- Integration with Rust: The framework is designed to work seamlessly with Rust, the primary language for writing Solana smart contracts. This allows developers to leverage Rust's features for testing.
- CLI Tools: Solana provides command-line interface (CLI) tools that facilitate the deployment and testing of smart contracts. These tools can be used to run tests directly from the terminal.
- Testnet Environment: Developers can use Solana's testnet to deploy and test their contracts in a live environment without the risk of losing real funds. This is crucial for testing interactions with other contracts and accounts.
- Documentation and Examples: Solana's official documentation includes examples and guidelines for writing tests, making it easier for developers to get started.
To effectively utilize Solana's testing framework, follow these steps:
- Set up your development environment with the necessary tools and libraries.
- Write unit tests using the chosen testing framework (e.g., Mocha or Jest).
- Use mock accounts and transactions to simulate various scenarios.
- Run tests using the CLI tools provided by Solana.
- Analyze test results and iterate on your smart contract code as needed.
By following these practices, developers can ensure that their Solana smart contracts are robust, efficient, and ready for deployment.
At Rapid Innovation, we understand the complexities involved in blockchain development, and we are here to guide you through every step of the process. Our expertise in AI and blockchain technology enables us to provide tailored solutions that enhance your project’s efficiency and effectiveness. By partnering with us, you can expect greater ROI through optimized development processes, reduced time-to-market, and improved contract reliability. Let us help you achieve your goals with confidence and precision.
4.2. Writing Your First Unit Test for a Solana Program
Writing unit tests for a Solana program is essential to ensure that your smart contracts function as intended. The Solana ecosystem provides tools like the Solana Web3.js library and the Anchor framework, which simplify the testing process.
To write your first unit test:
- Set up your development environment with Node.js and install necessary packages:
language="language-bash"npm install --save-dev @solana/web3.js @project-serum/anchor
- Create a test file in your project directory, typically under a
tests
folder, e.g.,my_program.test.js
. - Import the required libraries and set up your test environment:
language="language-javascript"const anchor = require('@project-serum/anchor');-a1b2c3-const { SystemProgram } = anchor.web3;
- Write a simple test case to check if your program can be deployed and initialized correctly:
language="language-javascript"describe('My Solana Program', () => {-a1b2c3- it('Initializes the program', async () => {-a1b2c3- const provider = anchor.Provider.local();-a1b2c3- anchor.setProvider(provider);-a1b2c3- const program = anchor.workspace.MyProgram;-a1b2c3--a1b2c3- const tx = await program.rpc.initialize();-a1b2c3- console.log("Transaction signature", tx);-a1b2c3- });-a1b2c3-});
- Run your tests using the command:
language="language-bash"anchor test
This basic structure allows you to build upon it by adding more complex tests as your program evolves.
4.3. Mocking Accounts and Signatures in Unit Tests
Mocking accounts and signatures is crucial for testing various scenarios without needing real accounts or transactions. This allows you to simulate different states and behaviors of your program.
To mock accounts and signatures:
- Use the
anchor
library to create mock accounts:
language="language-javascript"const mockAccount = anchor.web3.Keypair.generate();
- Set up the mock account in your test:
language="language-javascript"it('Mocks an account', async () => {-a1b2c3- const provider = anchor.Provider.local();-a1b2c3- anchor.setProvider(provider);-a1b2c3- const program = anchor.workspace.MyProgram;-a1b2c3--a1b2c3- await program.rpc.initialize({-a1b2c3- accounts: {-a1b2c3- myAccount: mockAccount.publicKey,-a1b2c3- user: provider.wallet.publicKey,-a1b2c3- systemProgram: SystemProgram.programId,-a1b2c3- },-a1b2c3- });-a1b2c3-});
- You can also mock signatures by using the
Keypair
class to generate a new keypair for testing:
language="language-javascript"const userKeypair = anchor.web3.Keypair.generate();
- Ensure that your tests check for expected outcomes based on the mocked data:
language="language-javascript"it('Checks the account state', async () => {-a1b2c3- const accountData = await program.account.myAccount.fetch(mockAccount.publicKey);-a1b2c3- assert.ok(accountData.initialized);-a1b2c3-});
4.4. Testing Different Scenarios and Edge Cases
Testing different scenarios and edge cases is vital to ensure your program handles unexpected inputs and conditions gracefully. This includes testing for invalid inputs, boundary conditions, and failure cases.
To effectively test these scenarios:
- Identify potential edge cases relevant to your program logic, such as:
- Invalid account states
- Exceeding maximum limits
- Unauthorized access attempts
- Write tests for each identified scenario:
language="language-javascript"it('Fails on unauthorized access', async () => {-a1b2c3- try {-a1b2c3- await program.rpc.someAction({-a1b2c3- accounts: {-a1b2c3- myAccount: mockAccount.publicKey,-a1b2c3- user: unauthorizedUser.publicKey,-a1b2c3- },-a1b2c3- });-a1b2c3- assert.fail("Expected error not received");-a1b2c3- } catch (err) {-a1b2c3- assert.ok(err.message.includes("Unauthorized"));-a1b2c3- }-a1b2c3-});
- Use assertions to validate that your program behaves as expected under these conditions:
language="language-javascript"it('Handles invalid input gracefully', async () => {-a1b2c3- const invalidInput = -1; // Example of invalid input-a1b2c3- await assert.rejects(async () => {-a1b2c3- await program.rpc.processInput(invalidInput);-a1b2c3- }, /Invalid input/);-a1b2c3-});
By thoroughly testing various scenarios and edge cases, you can ensure that your Solana program is robust and reliable, ready for deployment in a live environment.
At Rapid Innovation, we understand the importance of rigorous testing in the development of blockchain applications. Our expertise in AI and blockchain development ensures that your projects are not only innovative but also secure and efficient. By partnering with us, you can expect enhanced ROI through reduced development time, minimized errors, and a streamlined path to market. Let us help you achieve your goals effectively and efficiently.
5. Integration Testing for Solana Programs
At Rapid Innovation, we understand that integration testing is crucial for ensuring that Solana programs work correctly when combined. This process helps identify issues that may not be apparent during unit testing, ultimately leading to a more robust and reliable application.
5.1. Setting Up an Integration Test Environment
To effectively conduct integration testing for Solana programs, it is essential to set up a suitable environment. This involves several steps:
- Install Required Tools: Ensure you have the necessary tools installed, such as Rust, Solana CLI, and the Anchor framework.
- Create a Local Cluster: Use the Solana CLI to set up a local test validator. This allows you to simulate a Solana environment without incurring costs.
language="language-bash"solana-test-validator
- Configure Your Environment: Set your Solana CLI to point to the local cluster. This can be done by running:
language="language-bash"solana config set --url http://localhost:8899
- Deploy Your Programs: Compile and deploy your Solana programs to the local cluster. Use the following commands:
language="language-bash"anchor build-a1b2c3-anchor deploy
- Set Up Test Framework: Use a testing framework like Mocha or Jest to write your integration tests. Ensure you have the necessary dependencies installed.
- Write Test Cases: Create test cases that cover various scenarios, including edge cases. This will help ensure that your programs interact correctly.
- Run Tests: Execute your tests against the local cluster to verify that everything works as expected.
5.2. Testing Interactions Between Multiple Solana Programs
When multiple Solana programs interact, it’s essential to test these interactions to ensure they function correctly together. Here are steps to achieve this:
- Identify Interactions: Determine which programs will interact and the nature of their interactions (e.g., data sharing, token transfers).
- Set Up Mock Data: Create mock data that simulates real-world scenarios. This will help in testing how programs handle various inputs.
- Write Integration Tests: Develop integration tests that specifically focus on the interactions between the programs. This can include:
- Cross-Program Invocations: Test how one program calls another and handles the response.
- State Changes: Verify that state changes in one program are reflected in another as expected.
- Use Transaction Simulation: Leverage Solana's transaction simulation features to test how transactions involving multiple programs will behave without actually executing them.
- Monitor Logs and Events: During testing, monitor logs and events to catch any unexpected behavior or errors. This can be done using the Solana CLI or programmatically.
- Iterate and Refine: Based on the results of your tests, refine your programs and tests. This iterative process helps ensure robustness.
By following these steps, you can effectively set up an integration test environment and test interactions between multiple Solana programs, ensuring that they work seamlessly together. At Rapid Innovation, we are committed to helping you achieve greater ROI through our expertise in AI and Blockchain development. Partnering with us means you can expect enhanced efficiency, reduced risks, and a more streamlined path to achieving your business goals. Let us guide you through the complexities of integration testing and beyond, ensuring your projects are not only successful but also sustainable.
5.3. Simulating On-Chain Transactions in Tests
Simulating on-chain transactions is crucial for testing Solana smart contracts. It allows developers to validate the behavior of their programs without incurring transaction fees or affecting the live network.
- Use the Solana CLI or a testing framework like Anchor to set up your testing environment.
- Create a local test validator to simulate the Solana blockchain.
- Write test cases that mimic real-world scenarios, including successful transactions and edge cases.
Example code snippet for simulating a transaction:
language="language-javascript"const { Connection, Keypair, Transaction, SystemProgram } = require('@solana/web3.js');-a1b2c3--a1b2c3-const connection = new Connection('http://localhost:8899', 'confirmed');-a1b2c3-const payer = Keypair.generate();-a1b2c3--a1b2c3-async function simulateTransaction() {-a1b2c3- const transaction = new Transaction().add(-a1b2c3- SystemProgram.transfer({-a1b2c3- fromPubkey: payer.publicKey,-a1b2c3- toPubkey: Keypair.generate().publicKey,-a1b2c3- lamports: 1000,-a1b2c3- })-a1b2c3- );-a1b2c3--a1b2c3- const { value: simulationResult } = await connection.simulateTransaction(transaction, { accounts: [payer.publicKey] });-a1b2c3- console.log(simulationResult);-a1b2c3-}-a1b2c3--a1b2c3-simulateTransaction();
- Ensure to check the simulation results for errors or unexpected behavior.
- Use assertions in your tests to validate the expected outcomes.
5.4. Verifying Program Behavior Across Different Accounts
Verifying program behavior across different accounts is essential to ensure that your smart contract functions correctly regardless of the account state or ownership.
- Create multiple accounts with different states to test various scenarios.
- Use the Solana CLI or Anchor framework to deploy your program and interact with these accounts.
Steps to verify program behavior:
- Set up different accounts with varying balances and permissions.
- Execute transactions from these accounts to observe how the program responds.
- Check for correct state changes and error handling.
Example code snippet for testing different accounts:
language="language-javascript"const { Connection, Keypair, Transaction, SystemProgram } = require('@solana/web3.js');-a1b2c3--a1b2c3-async function testDifferentAccounts() {-a1b2c3- const connection = new Connection('http://localhost:8899', 'confirmed');-a1b2c3- const account1 = Keypair.generate();-a1b2c3- const account2 = Keypair.generate();-a1b2c3--a1b2c3- // Fund accounts-a1b2c3- await connection.requestAirdrop(account1.publicKey, 10000);-a1b2c3- await connection.requestAirdrop(account2.publicKey, 5000);-a1b2c3--a1b2c3- // Create a transaction from account1 to account2-a1b2c3- const transaction = new Transaction().add(-a1b2c3- SystemProgram.transfer({-a1b2c3- fromPubkey: account1.publicKey,-a1b2c3- toPubkey: account2.publicKey,-a1b2c3- lamports: 2000,-a1b2c3- })-a1b2c3- );-a1b2c3--a1b2c3- // Send transaction and verify results-a1b2c3- const signature = await connection.sendTransaction(transaction, [account1]);-a1b2c3- await connection.confirmTransaction(signature);-a1b2c3- console.log('Transaction successful:', signature);-a1b2c3-}-a1b2c3--a1b2c3-testDifferentAccounts();
- Validate the final balances of the accounts to ensure the transaction was processed correctly.
- Test edge cases, such as insufficient funds or unauthorized access.
6. Debugging Techniques for Solana Smart Contracts
Debugging Solana smart contracts can be challenging due to the asynchronous nature of blockchain transactions. However, several techniques can help identify and resolve issues effectively.
- Use logging: Implement logging within your smart contract to track the flow of execution and variable states. This can help pinpoint where things go wrong.
Example of logging in Rust:
language="language-rust"msg!("This is a log message: {:?}", variable);
- Utilize the Solana Explorer: After deploying your contract, use the Solana Explorer to view transaction details, including errors and program logs.
- Test locally: Use the local test validator to run your smart contracts in a controlled environment. This allows for rapid iteration and debugging without the need for network transactions.
- Leverage unit tests: Write comprehensive unit tests for your smart contracts to catch issues early in the development process. Use frameworks like Anchor to simplify testing.
- Analyze error messages: Pay close attention to error messages returned by the Solana runtime. They often provide valuable insights into what went wrong.
By employing these debugging techniques, developers can enhance the reliability and performance of their Solana smart contracts.
At Rapid Innovation, we understand the complexities involved in blockchain development. Our expertise in AI and blockchain technology enables us to provide tailored solutions that help clients achieve their goals efficiently and effectively. By partnering with us, you can expect greater ROI through optimized development processes, reduced time-to-market, and enhanced product reliability. Let us help you navigate the blockchain landscape and unlock the full potential of your projects.
6.1. Using Solana's Native Debugging Tools
At Rapid Innovation, we understand that effective debugging is crucial for the success of your blockchain projects. Solana provides several native debugging tools that can help developers identify issues in their programs. These tools are essential for ensuring that your smart contracts run smoothly and efficiently.
- Solana CLI: The command-line interface allows you to interact with the Solana blockchain. You can use it to deploy programs, check account balances, and view transaction details.
- Solana Explorer: This web-based tool provides a user-friendly interface to view transactions, accounts, and program logs. You can search for specific transactions and analyze their status.
- Debugging with
solana-test-validator
: This local validator simulates the Solana blockchain environment. You can run your programs in a controlled setting, making it easier to test and debug. - Using
solana logs
command: This command retrieves logs from the Solana cluster, allowing you to see detailed information about transactions and program execution.
To utilize these tools effectively, follow these steps:
- Install the Solana CLI and set up your environment.
- Use
solana-test-validator
to create a local testing environment. - Deploy your program using the CLI.
- Execute transactions and monitor logs using the
solana logs
command.
By leveraging these tools, our clients can significantly reduce development time and enhance the reliability of their applications, ultimately leading to greater ROI.
6.2. Implementing Logging and Error Handling in Your Programs
At Rapid Innovation, we emphasize the importance of effective logging and error handling for maintaining the reliability of your Solana programs. By implementing these practices, you can quickly identify and resolve issues.
- Use the
msg!
macro: This macro allows you to log messages at various points in your program. It can be helpful for tracking the flow of execution and identifying where errors occur. - Error handling with
Result
type: Utilize Rust'sResult
type to manage errors gracefully. This allows you to return meaningful error messages instead of panicking. - Custom error types: Define custom error types for your program. This provides more context about the nature of the error and helps in debugging.
- Log important state changes: Whenever a significant state change occurs, log it. This can include changes to account balances, program state, or any critical operations.
To implement logging and error handling, follow these steps:
- Define custom error types in your program.
- Use the
msg!
macro to log important events. - Implement the
Result
type for functions that may fail. - Test your program thoroughly to ensure that logging captures all necessary information.
By partnering with us, clients can expect a more robust application that minimizes downtime and enhances user experience, leading to improved customer satisfaction and retention.
6.3. Analyzing Transaction Logs and Error Messages
Analyzing transaction logs and error messages is vital for diagnosing issues in your Solana programs. Understanding these logs can help you pinpoint the source of errors and improve your code.
- Accessing transaction logs: Use the Solana Explorer or the CLI to access transaction logs. Look for specific transactions related to your program.
- Interpreting error messages: Solana provides detailed error messages that can help you understand what went wrong. Pay attention to the error codes and descriptions.
- Cross-referencing with code: When you encounter an error, cross-reference the logs with your code. This can help you identify the exact line or function where the issue occurred.
- Using community resources: Leverage community forums and documentation to understand common errors and their solutions. The Solana community is active and can provide valuable insights.
To analyze transaction logs effectively, follow these steps:
- Retrieve transaction logs using the Solana Explorer or CLI.
- Identify the error messages and codes present in the logs.
- Cross-reference these messages with your program's code.
- Consult community resources for additional context and solutions.
By utilizing our expertise in analyzing transaction logs, clients can enhance their debugging processes, leading to faster resolution of issues and a more efficient development cycle. This ultimately translates to a higher return on investment and a competitive edge in the market.
6.4. Debugging Cross-Program Invocations
Debugging cross-program invocations (CPI) in Solana can be challenging due to the asynchronous nature of the blockchain and the complexity of interactions between different programs. Here are some strategies to effectively debug CPIs:
- Understand the Call Flow:
- Familiarize yourself with how CPIs work in Solana. When one program calls another, it passes control and data, which can lead to unexpected behaviors if not handled correctly.
- Use Logging:
- Implement logging within your programs to track the flow of execution and the state of variables. This can help identify where things go wrong. Utilize the
msg!
macro to print debug messages to the Solana logs. - Error Handling:
- Ensure that your programs handle errors gracefully. Use the
Result
type to propagate errors back to the calling program, allowing for better debugging. - Test with Different Inputs:
- Create a variety of test cases with different inputs to see how your program behaves under various conditions. This can help uncover edge cases that may not be immediately obvious.
- Simulate Transactions:
- Use the Solana CLI or local test validators to simulate transactions. This allows you to see how your program interacts with others without incurring costs on the mainnet.
- Inspect Program State:
- Use tools like Solana Explorer or custom scripts to inspect the state of accounts before and after the invocation to understand the impact of the CPI.
7. Advanced Testing Strategies for Solana
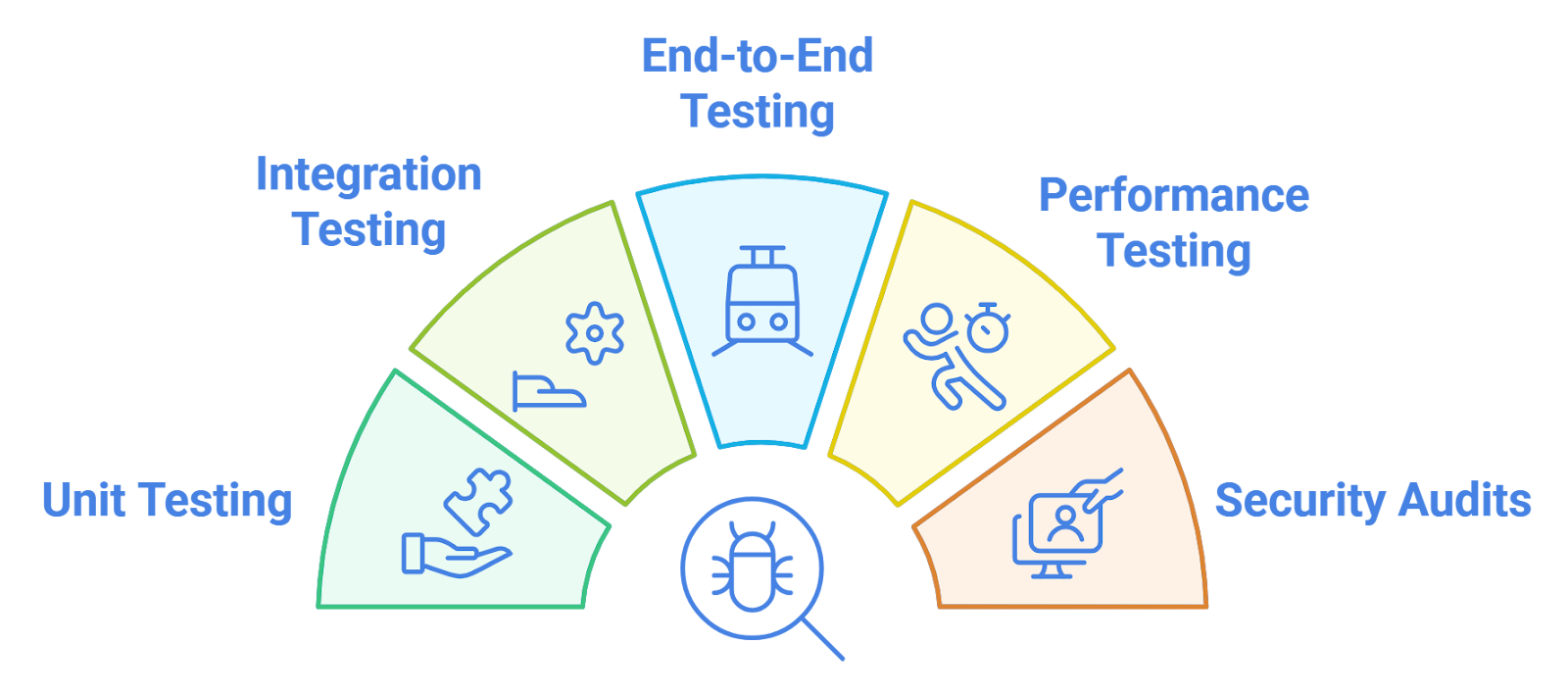
Advanced testing strategies are essential for ensuring the reliability and security of Solana programs. Here are some effective approaches:
- Unit Testing:
- Write unit tests for individual functions within your programs. This helps ensure that each component behaves as expected in isolation.
- Integration Testing:
- Test how different components of your program work together. This is particularly important for CPIs, as it verifies that the interactions between programs are functioning correctly.
- End-to-End Testing:
- Simulate real-world scenarios by testing the entire flow of your application. This includes deploying your program on a testnet and interacting with it as a user would.
- Performance Testing:
- Assess the performance of your program under load. Use tools to simulate multiple users interacting with your program simultaneously to identify bottlenecks.
- Security Audits:
- Conduct thorough security audits to identify vulnerabilities. This can include manual code reviews and automated tools to scan for common security issues.
7.1. Fuzz Testing Solana Programs
Fuzz testing is a powerful technique for discovering vulnerabilities in software by inputting random data into programs. In the context of Solana, fuzz testing can help identify edge cases and unexpected behaviors in your programs.
- Set Up a Fuzz Testing Environment:
- Use a local test validator to create a safe environment for fuzz testing without risking real assets.
- Choose a Fuzz Testing Tool:
- Select a fuzz testing tool compatible with Rust, such as
cargo-fuzz
, to automate the process of generating random inputs. - Define Input Constraints:
- Specify constraints for the inputs to ensure that the fuzzing process generates valid data that your program can process.
- Run Fuzz Tests:
- Execute the fuzz tests and monitor the program's behavior. Look for crashes, unexpected outputs, or any deviations from expected behavior.
- Analyze Results:
- Review the results of the fuzz tests to identify any vulnerabilities or bugs. Fix the issues and rerun the tests to ensure they are resolved.
- Integrate into CI/CD:
- Incorporate fuzz testing into your continuous integration and deployment pipeline to ensure ongoing security and reliability as your program evolves.
At Rapid Innovation, we understand the complexities of blockchain development and the importance of robust testing and debugging strategies, including debugging cross-program invocations. By partnering with us, clients can leverage our expertise to enhance their development processes, ultimately leading to greater ROI through reduced downtime, improved security, and more efficient program interactions. Our tailored solutions ensure that your projects are not only successful but also scalable and secure, allowing you to focus on your core business objectives.
7.2. Property-Based Testing for Robust Smart Contracts
At Rapid Innovation, we understand that property-based testing is a vital technique for ensuring the reliability of smart contracts. This method emphasizes verifying the properties of contracts rather than merely testing specific inputs, which helps guarantee that the contract behaves as expected across a diverse range of conditions.
- Key Properties to Test:
- Determinism: The same input should always produce the same output.
- Invariants: Certain conditions should always hold true throughout the contract's lifecycle.
- Safety: The contract should not allow invalid states or transitions.
- Tools for Property-Based Testing:
- QuickCheck: A popular tool in Haskell that can be adapted for smart contracts.
- Hypothesis: A Python library that allows for property-based testing.
- Fuzz Testing: Randomly generates inputs to discover edge cases.
- Steps to Implement Property-Based Testing:
- Define the properties you want to test.
- Use a property-based testing tool to generate random inputs.
- Run the tests and analyze the results to identify any failures.
- Refine the contract based on the findings and retest.
- Smart Contract Testing Tools: Utilizing smart contract testing tools can enhance the property-based testing process, ensuring comprehensive coverage of various scenarios.
7.3. Stress Testing Your Programs Under High Load
Stress testing is crucial for understanding how smart contracts perform under extreme conditions. At Rapid Innovation, we help clients identify bottlenecks and ensure that their contracts can handle high transaction volumes effectively.
- Objectives of Stress Testing:
- Determine the maximum load the contract can handle.
- Identify performance degradation under high load.
- Ensure that the contract remains functional during peak usage.
- Tools for Stress Testing:
- Gatling: A powerful tool for load testing web applications, which can be adapted for smart contracts.
- Apache JMeter: A widely used tool for performance testing that can simulate multiple users.
- Locust: A scalable load testing tool that allows for writing tests in Python.
- Steps to Conduct Stress Testing:
- Define the load conditions (e.g., number of transactions per second).
- Set up the testing environment with the necessary tools.
- Simulate the load on the smart contract.
- Monitor performance metrics such as response time and error rates.
- Analyze the results to identify any weaknesses or failures.
- Test Smart Contract Locally: Before deploying, it is essential to test smart contracts locally to ensure they can handle stress effectively.
7.4. Security Testing and Auditing Solana Smart Contracts
Security testing and auditing are paramount for ensuring the integrity and safety of smart contracts on the Solana blockchain. At Rapid Innovation, we recognize the financial implications of vulnerabilities, making thorough testing essential for our clients.
- Common Security Vulnerabilities:
- Reentrancy Attacks: Occur when a contract calls another contract and allows it to call back before the first call is complete.
- Integer Overflow/Underflow: Errors that occur when arithmetic operations exceed the maximum or minimum limits.
- Access Control Issues: Improperly configured permissions can lead to unauthorized access.
- Tools for Security Testing:
- MythX: A comprehensive security analysis tool for Ethereum and EVM-compatible smart contracts.
- Slither: A static analysis tool that detects vulnerabilities in Solidity code.
- Securify: A tool that checks smart contracts against a set of security properties.
- Steps for Security Testing and Auditing:
- Conduct a code review to identify potential vulnerabilities.
- Use automated tools to perform static and dynamic analysis.
- Test the contract under various attack scenarios.
- Document findings and recommend fixes.
- Retest the contract after implementing security measures.
- Smart Contract Penetration Testing: Incorporating smart contract penetration testing can help identify vulnerabilities that may not be caught through standard testing methods.
By implementing these testing strategies, including smart contract unit testing and utilizing solidity testing tools, Rapid Innovation empowers developers to enhance the robustness, performance, and security of their smart contracts. This ultimately leads to a more reliable and trustworthy blockchain ecosystem, ensuring that our clients achieve greater ROI and operational efficiency. Partnering with us means you can expect a comprehensive approach to development and consulting solutions that align with your business goals.
8. Continuous Integration and Deployment for Solana Projects
At Rapid Innovation, we understand that Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in modern software development, particularly for blockchain projects like those built on Solana. These practices not only streamline the development process but also significantly reduce errors, ensuring that code changes are automatically tested and deployed. By partnering with us, clients can leverage our expertise to implement these practices effectively, leading to greater efficiency and a higher return on investment (ROI).
8.1. Setting Up CI/CD Pipelines for Solana Development
Setting up a CI/CD pipeline for Solana projects involves several steps to ensure that your code is consistently integrated and deployed. Here’s how we can assist you in this process:
- Choose a CI/CD Tool: We help you select a CI/CD tool that best supports your development environment. Popular options include GitHub Actions, GitLab CI, and CircleCI, and we guide you in choosing the right one for your needs.
- Create a Repository: Our team will assist you in setting up a Git repository for your Solana project, ensuring that your code is stored and versioned correctly.
- Define Your Pipeline: We will work with you to create a configuration file (e.g.,
.yml
for GitHub Actions) that outlines the steps in your CI/CD process, specifying the build, test, and deployment stages. - Install Dependencies: In your pipeline configuration, we will include steps to install necessary dependencies for your Solana project, typically involving Rust and Solana CLI tools.
- Build the Project: Our experts will add a step to compile your Solana program, ensuring that your code is syntactically correct and executable.
- Deploy to Devnet/Testnet: After a successful build, we will configure your pipeline to deploy the code to Solana's Devnet or Testnet, allowing you to test your application in a live environment without affecting the mainnet.
- Monitor and Notify: We will set up notifications for build failures or deployment issues, utilizing tools like email, Slack, or other communication platforms to keep you informed.
Example of a simple GitHub Actions workflow for a Solana project:
language="language-yaml"name: CI/CD for Solana Project-a1b2c3--a1b2c3-on:-a1b2c3- push:-a1b2c3- branches:-a1b2c3- - main-a1b2c3--a1b2c3-jobs:-a1b2c3- build:-a1b2c3- runs-on: ubuntu-latest-a1b2c3- steps:-a1b2c3- - name: Checkout code-a1b2c3- uses: actions/checkout@v2-a1b2c3--a1b2c3- - name: Set up Rust-a1b2c3- uses: actions-rs/toolchain@v1-a1b2c3- with:-a1b2c3- toolchain: stable-a1b2c3--a1b2c3- - name: Install Solana CLI-a1b2c3- run: sh -c "$(curl -sSfL https://release.solana.com/v1.9.0/install)"-a1b2c3--a1b2c3- - name: Build the project-a1b2c3- run: cargo build --release-a1b2c3--a1b2c3- - name: Deploy to Devnet-a1b2c3- run: solana deploy target/release/your_program.so --url https://api.devnet.solana.com
8.2. Automating Tests in Your Build Process
Automating tests in your CI/CD pipeline is crucial for maintaining code quality and ensuring that new changes do not introduce bugs. Here’s how we can help you incorporate automated testing into your build process:
- Write Unit Tests: Our team will assist you in developing unit tests for your Solana program using Rust’s built-in testing framework, ensuring that all critical functionalities are covered.
- Integrate Testing in CI/CD: We will modify your CI/CD pipeline configuration to include a testing step that runs your unit tests after the build step.
- Use Test Frameworks: We recommend and help implement testing frameworks like
cargo test
for Rust, allowing you to run tests easily and obtain detailed reports. - Test Coverage: Our experts will implement test coverage tools to analyze how much of your code is tested, helping to identify untested areas that may need attention.
- Fail Fast: We will configure your pipeline to fail the build if any tests do not pass, ensuring that only code that meets quality standards is deployed.
Example of adding a test step to the previous GitHub Actions workflow:
language="language-yaml"- name: Run tests-a1b2c3- run: cargo test
By following these steps, Rapid Innovation can effectively set up CI/CD pipelines for your Solana projects, ensuring that your development process is efficient, reliable, and scalable. Partnering with us means you can expect enhanced productivity, reduced time-to-market, and ultimately, a greater ROI on your blockchain initiatives. Let us help you achieve your goals efficiently and effectively. For more insights on smart contracts, check out our guide to Master BSC Smart Contracts: Ultimate Guide to Development, Deployment & DeFi Integration.
8.3. Deploying and Upgrading Programs Safely

Deploying and upgrading programs in a blockchain environment like Solana requires meticulous planning to ensure both security and functionality. At Rapid Innovation, we understand the intricacies involved in this process and are here to guide you through it. Here are key considerations that we emphasize to our clients:
- Use Version Control: We recommend maintaining a version control system (like Git) to track changes in your codebase. This practice allows you to revert to previous versions if necessary, ensuring that your development process remains agile and responsive.
- Test Thoroughly: Before deployment, we conduct extensive testing in a local or testnet environment. This proactive approach helps identify bugs and performance issues without risking the integrity of the main network, ultimately saving you time and resources.
- Implement Upgradeable Contracts: Our team utilizes Solana's upgradeable program architecture, allowing you to deploy new versions of your program while retaining the state of the previous version. This flexibility is crucial for maintaining continuity in your operations.
- Use a Multi-Signature Wallet: For deploying and upgrading programs, we advocate the use of a multi-signature wallet. This requires multiple approvals before changes are made, adding an essential layer of security to your deployment process.
- Monitor Post-Deployment: After deployment, we continuously monitor the program for any unexpected behavior or performance issues. Our expertise in tools like Solana Explorer enables us to track transactions and program interactions effectively.
- Rollback Plan: We always ensure that you have a rollback plan in place in case the new deployment introduces critical issues. This could involve reverting to the last stable version of the program, safeguarding your operations against unforeseen challenges.
9. Performance Optimization and Benchmarking
Performance optimization is vital for ensuring that Solana programs run efficiently and effectively. At Rapid Innovation, we employ a range of strategies to enhance performance for our clients:
- Profile Your Code: We utilize profiling tools to analyze the performance of your program, identifying which functions consume the most resources and optimizing them for better efficiency.
- Optimize Data Structures: Our team helps you choose the right data structures for your program. For instance, using arrays instead of linked lists can significantly improve access times, leading to faster execution.
- Reduce Transaction Size: We focus on minimizing the size of transactions by only including necessary data. Smaller transactions are processed faster on the Solana network, enhancing overall performance.
- Batch Processing: Where possible, we implement batch processing to combine multiple operations into a single transaction. This approach reduces the overhead of multiple transactions and can lead to substantial performance gains.
- Use Efficient Algorithms: Our experts implement algorithms that are optimized for performance. For example, using a hash map for lookups can significantly reduce time complexity compared to linear searches.
- Benchmark Regularly: We conduct regular benchmarking of your program against previous versions and competitors. This practice helps identify areas for improvement and ensures that you are consistently meeting performance goals.
9.1. Identifying Performance Bottlenecks in Solana Programs
Identifying performance bottlenecks is essential for optimizing Solana programs. Here are steps that we take to effectively pinpoint these issues:
- Analyze Transaction Latency: We monitor the time it takes for transactions to be confirmed. High latency can indicate bottlenecks in your program, and we address these promptly.
- Use Logging: Our team implements logging to track the execution time of different functions within your program. This data helps us identify which parts of the code are slowing down performance.
- Monitor Resource Usage: We keep a close eye on CPU and memory usage during program execution. High resource consumption can indicate inefficiencies in your code, and we work to optimize these areas.
- Test Under Load: We simulate high-load scenarios to assess how your program performs under stress. This testing can reveal bottlenecks that may not be apparent under normal conditions.
- Review Smart Contract Logic: Our experts examine the logic of your smart contracts for inefficiencies. Complex logic can lead to longer execution times and higher costs, and we strive to simplify and optimize this logic.
- Utilize Profiling Tools: We leverage profiling tools specifically designed for Solana to gain insights into performance metrics. These tools provide detailed reports on function execution times and resource usage, enabling us to make informed improvements.
By following these guidelines, Rapid Innovation ensures that your Solana programs are deployed safely and optimized for performance. This commitment ultimately leads to a better user experience and more efficient operations, helping you achieve greater ROI and meet your business goals effectively. Partnering with us means you can expect enhanced security, improved performance, and a dedicated team focused on your success.
9.2. Optimizing Compute Unit Consumption
At Rapid Innovation, we understand that optimizing compute unit consumption is crucial for enhancing the efficiency of your Solana smart contracts. Each transaction on Solana consumes compute units, and minimizing this consumption can lead to lower transaction costs and improved performance, ultimately driving greater ROI for your business.
h4. Strategies for Optimization
- Efficient Algorithms: We recommend using algorithms with lower time complexity. For example, prefer O(n log n) algorithms over O(n^2) when processing large datasets to ensure faster execution and reduced costs.
- Data Structures: Choosing appropriate data structures is vital. For instance, using a hashmap can significantly reduce lookup times compared to arrays, enhancing overall performance.
- Batch Processing: Instead of processing transactions one by one, we advocate for batching them together. This approach reduces the overhead of multiple transactions and optimizes compute unit usage, leading to cost savings.
- Avoid Unnecessary Computations: Our team will help you identify and eliminate redundant calculations. Caching results of expensive computations when possible can further enhance efficiency.
- Use Native Types: We encourage utilizing Solana's native types and avoiding complex data structures that require additional serialization and deserialization, which can slow down performance.
- Limit State Changes: Minimizing the number of state changes in your contract is essential. Each state change consumes compute units, so reducing them can lead to significant savings.
- Profile Your Code: Our experts utilize profiling tools to identify bottlenecks in your smart contract. We focus on optimizing compute unit consumption to enhance performance.
9.3. Benchmarking Your Smart Contract's Performance
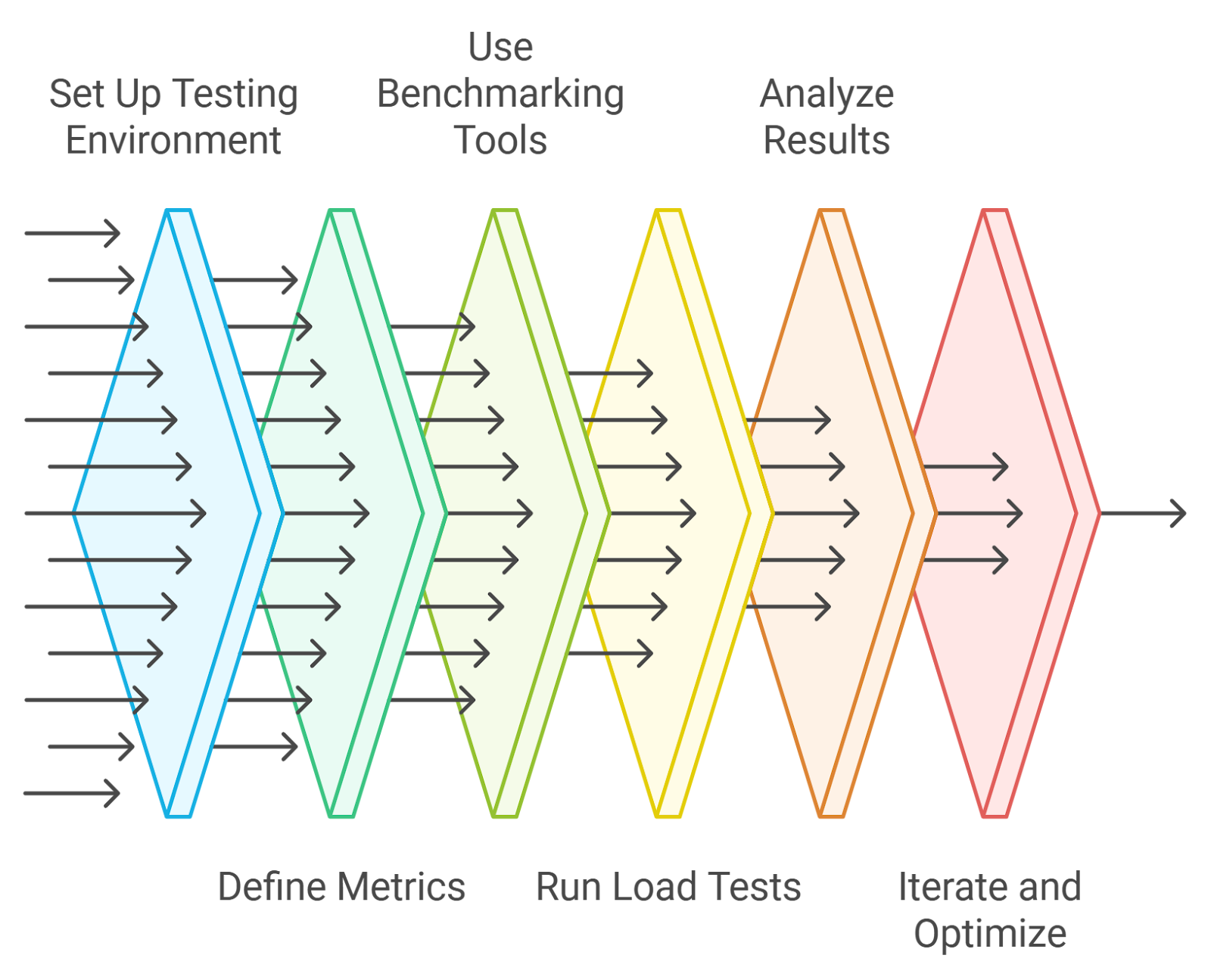
Benchmarking is essential to understand how your smart contract performs under various conditions. It helps identify performance bottlenecks and ensures that your contract meets the required standards, allowing you to achieve your business goals effectively.
h4. Steps for Effective Benchmarking
- Set Up a Testing Environment: We assist in creating a controlled environment that mimics the production environment as closely as possible to ensure accurate results.
- Define Metrics: Our team will help you determine what metrics to measure, such as transaction throughput, latency, and compute unit consumption, to gauge performance effectively.
- Use Benchmarking Tools: We leverage tools like Solana's built-in benchmarking utilities or third-party libraries to automate the benchmarking process, saving you time and resources.
- Run Load Tests: Simulating different loads on your smart contract allows us to see how it performs under stress, helping identify how it behaves with varying transaction volumes.
- Analyze Results: We collect and analyze the data from your benchmarks, looking for patterns and identifying areas for improvement to enhance performance.
- Iterate and Optimize: Based on our findings, we make necessary adjustments to your smart contract and re-benchmark to ensure performance has improved.
10. Troubleshooting Common Solana Development Issues
While developing on Solana, you may encounter various issues. Our expertise allows us to address these common problems effectively, ensuring a smooth development process.
h4. Common Issues and Solutions
- Transaction Failures: If transactions are failing, we will check for:
- Insufficient funds in the account.
- Incorrect program IDs or account addresses.
- Exceeding compute unit limits.
- Program Deployment Issues: If you face issues deploying your program, we ensure:
- You are using the correct Solana CLI commands.
- Your program is compiled correctly and matches the expected architecture.
- Account Data Mismatches: If you encounter data mismatches, we will:
- Double-check the serialization and deserialization of account data.
- Ensure that the data structure used in the program matches the expected format.
- Performance Bottlenecks: If your smart contract is slow, we will:
- Profile your code to identify slow functions.
- Optimize compute unit consumption as discussed earlier.
- Network Connectivity Issues: If you experience connectivity problems, we will:
- Check your internet connection and ensure you are connected to the correct Solana cluster.
- Use Solana's status page to check for network outages.
By partnering with Rapid Innovation, you can optimize your smart contracts, benchmark their performance effectively, and troubleshoot common issues that arise during Solana development. Our expertise ensures that you achieve your goals efficiently and effectively, leading to greater ROI for your business.
10.1. Resolving Account and Instruction Errors
At Rapid Innovation, we understand that account and instruction errors can stem from various sources, including user input mistakes, system misconfigurations, or network issues. Our expertise allows us to help clients effectively resolve these errors through a structured approach:
- Identify the Error:
- We assist in reviewing error messages and logs to pinpoint the issue.
- Our team checks for common issues such as incorrect account credentials or misconfigured settings, ensuring a swift resolution.
- Validate User Input:
- We implement robust input validation checks to ensure that all user inputs are correctly formatted and within expected parameters, preventing invalid data from being processed.
- Check System Configuration:
- Our experts verify that system settings align with the required configurations for account management, ensuring that all necessary services are running and accessible.
- Network Connectivity:
- We conduct thorough tests of network connections to ensure that applications can communicate with external services, utilizing tools like ping or traceroute to diagnose connectivity issues.
- Consult Documentation:
- Our team refers to the application’s documentation for troubleshooting steps specific to account and instruction errors, looking for known issues or FAQs that may provide insights into resolving the problem.
10.2. Debugging Serialization and Deserialization Problems

Serialization and deserialization are critical processes in data handling, especially when transferring data between systems. At Rapid Innovation, we help clients debug these issues to prevent data corruption or loss:
- Understand the Data Format:
- We ensure that the data being serialized and deserialized is in the correct format (e.g., JSON, XML) and use schema validation tools to confirm that the data structure matches expectations.
- Log Serialization/Deserialization Events:
- Our team implements logging to capture the state of data before and after serialization/deserialization, reviewing logs for discrepancies or errors during these processes.
- Test with Sample Data:
- We create controlled test cases with known data to isolate serialization and deserialization issues, comparing the output of serialized data against the original input to identify inconsistencies.
- Use Debugging Tools:
- We utilize advanced debugging tools or libraries that can help visualize the serialization process, ensuring data integrity through thorough testing.
- Check for Version Compatibility:
- Our experts ensure that the serialization format is compatible with the version of the libraries or frameworks being used, reviewing release notes for any breaking changes that may affect serialization.
10.3. Handling Program Upgrades and Data Migration
When upgrading software or migrating data, careful planning is essential to avoid data loss and ensure system integrity. Rapid Innovation provides comprehensive support to effectively manage these processes:
- Create a Backup:
- We emphasize the importance of backing up existing data before initiating an upgrade or migration, using reliable backup solutions to ensure data can be restored if needed.
- Plan the Upgrade/Migration:
- Our team develops a detailed plan outlining the steps involved in the upgrade or migration process, identifying potential risks and creating mitigation strategies. This includes considerations for data migration, such as cloud data migration services and database migration to cloud.
- Test the Upgrade/Migration:
- We conduct trial runs in a staging environment to identify any issues before going live, validating that all functionalities work as expected post-upgrade/migration. This may involve using services like AWS database migration service or Azure database migration service.
- Monitor the Process:
- Our experts keep a close eye on system performance and error logs during the upgrade/migration, being prepared to roll back changes if critical issues arise. We also ensure that data migration on cloud platforms is executed smoothly.
- Communicate with Stakeholders:
- We ensure that users and stakeholders are informed about the upgrade/migration schedule and potential impacts, providing support channels for users to report issues or seek assistance. This includes guidance on data migration services and the migration of database to AWS or Azure.
By partnering with Rapid Innovation, clients can expect a streamlined approach to resolving account and instruction errors, debugging serialization and deserialization problems, and managing program upgrades and data migrations. Our commitment to efficiency and effectiveness translates into greater ROI for your organization, especially when leveraging advanced solutions like AWS data migration and cloud database migration.
11. Best Practices for Maintaining Solana Smart Contracts
11.1. Implementing Proper Version Control
At Rapid Innovation, we understand that version control is crucial for managing changes in your Solana smart contracts. It allows developers to track modifications, collaborate effectively, and revert to previous versions if necessary. Here are some best practices for implementing version control that we recommend to our clients:
- Use Git: Git is the most widely used version control system. It allows you to create branches for new features or bug fixes, making it easier to manage changes without affecting the main codebase.
- Semantic Versioning: Adopt semantic versioning (e.g., MAJOR.MINOR.PATCH) to communicate the nature of changes. This helps users understand the impact of updates.
- Commit Messages: Write clear and descriptive commit messages. This practice aids in understanding the history of changes and the rationale behind them.
- Branching Strategy: Implement a branching strategy like Git Flow or GitHub Flow. This helps in organizing work and managing releases effectively.
- Regular Merges: Regularly merge changes from the main branch into feature branches to minimize conflicts and ensure compatibility.
- Tagging Releases: Use tags to mark specific points in your repository’s history as important, such as releases or stable versions. This makes it easier to roll back to a known good state if needed.
11.2. Documenting Your Testing and Debugging Processes
Documenting your testing and debugging processes is essential for maintaining the integrity of your Solana smart contracts. It ensures that all team members are on the same page and helps in identifying issues quickly. Here are some key practices that we advocate for our clients:
- Testing Frameworks: Utilize testing frameworks like Anchor or Mocha for writing unit tests. This ensures that your smart contracts behave as expected.
- Test Coverage: Aim for high test coverage to ensure that most of your code is tested. Tools like Istanbul can help measure coverage.
- Automated Testing: Implement continuous integration (CI) tools to automate testing. This ensures that tests are run every time changes are made, catching issues early.
- Debugging Tools: Use debugging tools like Solana Explorer or the Solana CLI to trace transactions and identify issues in your smart contracts.
- Documentation: Maintain a detailed log of your testing and debugging processes. Include:
- Test cases and their expected outcomes
- Steps taken to reproduce bugs
- Solutions implemented to fix issues
- Code Reviews: Conduct regular code reviews to ensure that testing and debugging practices are followed. This promotes knowledge sharing and improves code quality.
By following these best practices, you can ensure that your Solana smart contracts remain robust, maintainable, and efficient over time. At Rapid Innovation, we are committed to helping our clients achieve greater ROI through effective development and consulting solutions tailored to their specific needs. Partnering with us means you can expect enhanced efficiency, reduced risks, and a streamlined approach to your blockchain projects. Let us help you navigate the complexities of smart contract development and maintenance, ensuring your success in the ever-evolving digital landscape.
11.3. Conducting Regular Code Reviews and Audits
At Rapid Innovation, we understand that regular code reviews and audits, including code quality audit, ruby code audit, and code reviews and audits, are essential practices in software development, particularly in the context of blockchain and decentralized finance (DeFi) protocols. These processes are vital for identifying vulnerabilities, ensuring code quality, and maintaining compliance with best practices, ultimately helping our clients achieve their goals efficiently and effectively.
Benefits of Regular Code Reviews and Audits:
- Early Detection of Bugs: Our code review process allows developers to catch bugs early in the development cycle, significantly reducing the cost and effort required for fixes later. This proactive approach translates into greater ROI for our clients.
- Improved Code Quality: By promoting adherence to coding standards and best practices, regular reviews lead to cleaner, more maintainable code. This not only enhances performance but also reduces long-term maintenance costs.
- Knowledge Sharing: Code reviews facilitate knowledge transfer among team members, enhancing overall team competency and collaboration. This collective expertise ensures that our clients benefit from a well-rounded development team.
- Security Assurance: Our audits help identify security vulnerabilities, which is crucial in DeFi where financial assets are at stake. By ensuring robust security measures, we protect our clients' investments and build trust with their users.
Steps to Conduct Effective Code Reviews:
- Establish Clear Guidelines: We define what aspects of the code should be reviewed, such as functionality, performance, security, and style, ensuring a comprehensive evaluation.
- Use Code Review Tools: We implement tools like GitHub, GitLab, or Bitbucket to streamline the review process, making it efficient and effective.
- Set a Review Schedule: Regularly scheduled code reviews are integrated into the development workflow, ensuring they are a consistent part of our process.
- Encourage Constructive Feedback: We foster an environment where team members can provide and receive feedback positively, promoting a culture of continuous improvement.
- Document Findings: Keeping a record of issues found during reviews and tracking their resolution ensures accountability and transparency.
12. Case Studies: Real-World Testing and Debugging Scenarios
At Rapid Innovation, we leverage case studies to provide valuable insights into the practical challenges faced during testing and debugging in software development. These real-world examples illustrate how our teams have successfully navigated complex issues, particularly in high-stakes environments like DeFi.
Key Aspects of Case Studies:
- Real-World Context: Our case studies reflect actual scenarios, making them relatable and applicable to similar situations faced by our clients.
- Problem-Solving Approaches: They showcase various strategies employed to tackle specific challenges, offering lessons learned that can be applied to future projects.
- Outcome Analysis: Evaluating the results of debugging efforts helps our teams understand what worked and what didn’t, allowing us to refine our processes continuously.
12.1. Debugging a Complex DeFi Protocol on Solana
Debugging a complex DeFi protocol on Solana can be particularly challenging due to the unique architecture and performance characteristics of the Solana blockchain. Here’s how we approach it effectively:
Challenges in Debugging on Solana:
- High Throughput: Solana’s architecture allows for high transaction speeds, which can complicate debugging as issues may arise from race conditions or timing.
- Complex Interactions: DeFi protocols often involve multiple smart contracts interacting with each other, making it difficult to trace the source of an error.
- Limited Tooling: While Solana has made strides in developer tooling, it may not be as mature as other ecosystems, requiring developers to rely on custom solutions.
Steps to Debug a DeFi Protocol on Solana:
- Reproduce the Issue: We start by replicating the bug in a controlled environment to understand its behavior.
- Utilize Logging: Our team implements extensive logging within the smart contracts to capture state changes and transaction details, providing critical insights.
- Analyze Transaction History: We use Solana’s transaction explorer to review the history of transactions related to the protocol, helping to pinpoint issues.
- Test in Isolation: Isolating components of the protocol allows us to identify which part is causing the issue, streamlining the debugging process.
- Leverage Community Resources: We engage with the Solana developer community for insights and potential solutions to common problems, ensuring we stay at the forefront of industry knowledge.
By conducting regular code reviews and audits, including code quality audit and ruby code audit, and learning from real-world case studies, Rapid Innovation enhances the reliability and security of DeFi protocols, ultimately leading to a more robust ecosystem for our clients. Partnering with us means investing in a future where your projects are not only successful but also secure and efficient.
12.2. Optimizing a High-Throughput NFT Marketplace
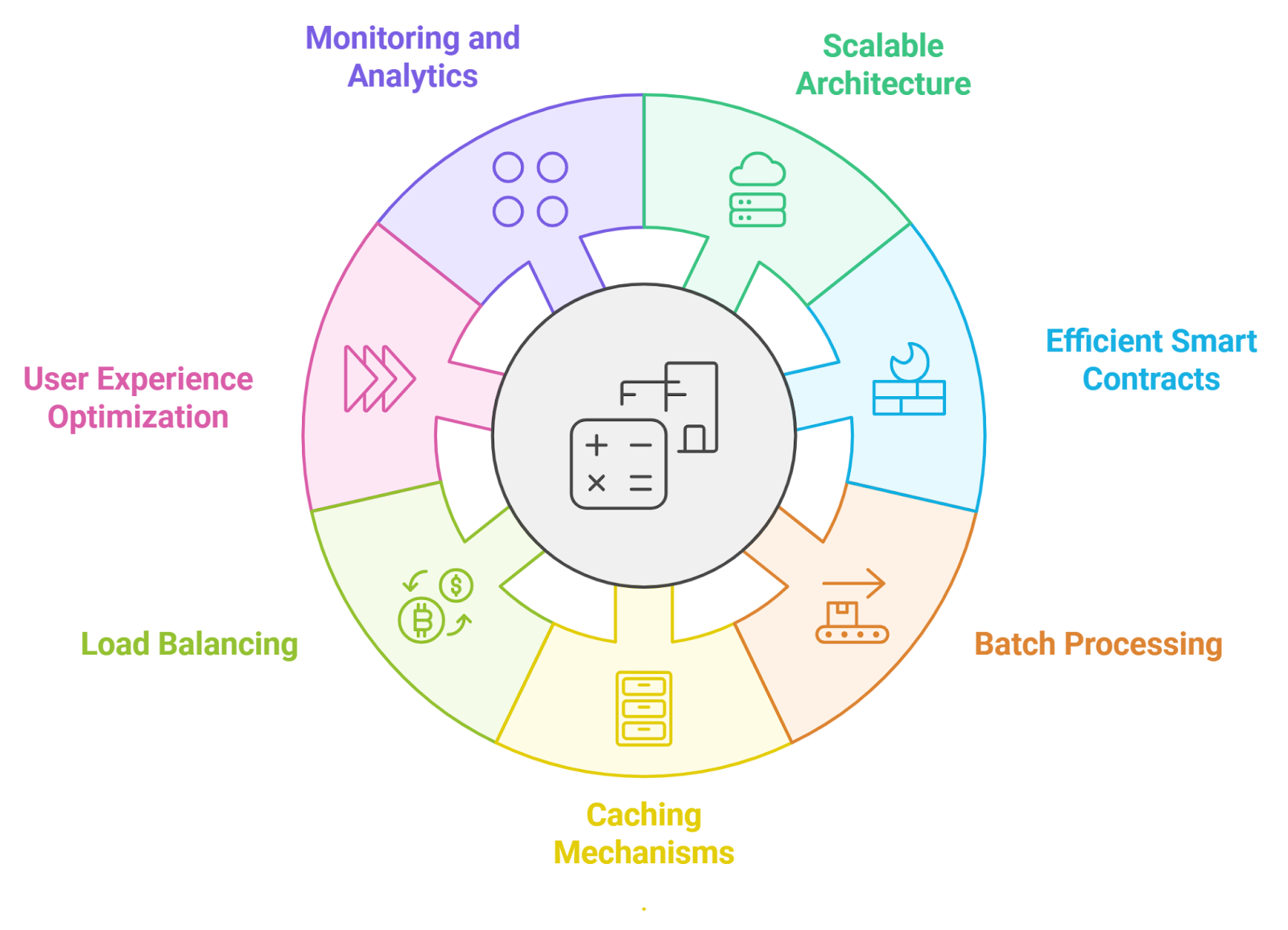
Creating a high-throughput NFT marketplace requires careful consideration of various factors to ensure that it can handle a large volume of transactions efficiently. Here are some strategies to optimize such a marketplace:
- Scalable Architecture: Utilize a microservices architecture to allow different components of the marketplace to scale independently. This can help manage increased loads without affecting the entire system.
- Efficient Smart Contracts: Write optimized smart contracts to minimize gas fees and execution time. Use tools like Solana's Rust SDK to create efficient code that reduces the computational burden on the network.
- Batch Processing: Implement batch processing for transactions. Instead of processing each transaction individually, group them together to reduce the number of interactions with the blockchain.
- Caching Mechanisms: Use caching strategies to store frequently accessed data off-chain. This can significantly reduce the load on the blockchain and improve response times for users.
- Load Balancing: Distribute incoming traffic across multiple servers to prevent any single point of failure. This ensures that the marketplace remains responsive even during peak usage times.
- User Experience Optimization: Enhance the user interface and experience to facilitate quicker transactions. This includes simplifying the onboarding process and providing clear feedback during transactions.
- Monitoring and Analytics: Implement monitoring tools to track performance metrics and user behavior. This data can help identify bottlenecks and areas for improvement.
12.3. Securing a Multi-Signature Wallet Implementation
A multi-signature wallet adds an extra layer of security by requiring multiple private keys to authorize a transaction. Here are steps to implement a secure multi-signature wallet:
- Define Signer Requirements: Determine how many signatures are required for a transaction to be valid. Common configurations include 2-of-3 or 3-of-5 setups.
- Choose a Secure Development Framework: Use established libraries and frameworks for wallet development, such as Solana's Wallet Adapter or other reputable libraries.
- Implement Key Management: Ensure that private keys are stored securely. Use hardware wallets or secure key management solutions to protect against unauthorized access.
- Create a User-Friendly Interface: Design an intuitive interface that allows users to easily manage their multi-signature wallets. Provide clear instructions on how to sign transactions.
- Test Thoroughly: Conduct extensive testing to identify vulnerabilities. Use tools like MythX or Slither to analyze smart contracts for potential security flaws.
- Regular Audits: Schedule regular security audits to ensure that the wallet implementation remains secure against emerging threats.
- Educate Users: Provide educational resources to users about the importance of multi-signature wallets and best practices for maintaining security.
13. Conclusion and Future Trends in Solana Smart Contract Testing
As the blockchain ecosystem continues to evolve, the importance of robust smart contract testing cannot be overstated. Future trends in Solana smart contract testing may include:
- Increased Automation: The use of automated testing tools will likely grow, allowing developers to quickly identify issues and ensure code quality.
- Integration of AI: Artificial intelligence may play a role in predicting vulnerabilities and optimizing smart contract performance.
- Focus on Interoperability: As more blockchains emerge, testing frameworks will need to adapt to ensure compatibility across different platforms.
- Enhanced Security Protocols: With the rise of DeFi and NFTs, security will remain a top priority, leading to the development of more sophisticated testing methodologies.
- Community-Driven Testing: Open-source testing frameworks and community contributions will likely become more prevalent, fostering collaboration and innovation in the space.
At Rapid Innovation, we understand the complexities involved in developing and optimizing blockchain solutions. Our expertise in AI and blockchain technology enables us to provide tailored consulting and development services that help clients achieve their goals efficiently and effectively. By partnering with us, clients can expect greater ROI through optimized performance, enhanced security, and improved user experiences. Let us help you navigate the evolving landscape of blockchain technology and unlock the full potential of your projects.
13.1. Emerging Tools and Frameworks for Solana Testing
The Solana blockchain is gaining traction, leading to the development of various tools and frameworks aimed at enhancing testing processes. These tools help developers ensure the reliability and security of their smart contracts and decentralized applications (dApps).
- Anchor: A framework that simplifies the development of Solana smart contracts. It provides a set of tools for building, testing, and deploying contracts efficiently.
- Solana CLI: The command-line interface for Solana allows developers to interact with the blockchain, deploy programs, and run tests directly from the terminal.
- Solana Test Validator: A local testing environment that simulates the Solana blockchain, enabling developers to test their applications without incurring transaction fees or waiting for confirmations.
- Phantom Wallet: While primarily a wallet, it also offers a testing environment for developers to interact with their dApps and test user experiences.
- Solana Explorer: A tool for monitoring transactions and program interactions on the Solana blockchain, useful for debugging and performance analysis.
These tools collectively enhance the testing landscape for Solana, making it easier for developers to build robust applications. At Rapid Innovation, we leverage these tools to ensure that our clients' projects are not only functional but also secure and efficient, ultimately leading to greater ROI.
13.2. The Role of AI and Machine Learning in Smart Contract Debugging
Artificial Intelligence (AI) and Machine Learning (ML) are becoming increasingly important in the realm of smart contract development, particularly in debugging. These technologies can significantly reduce the time and effort required to identify and fix issues in smart contracts.
- Automated Testing: AI can automate the testing process, allowing for more comprehensive coverage of potential vulnerabilities. This reduces the likelihood of human error during testing.
- Anomaly Detection: Machine learning algorithms can analyze transaction patterns and detect anomalies that may indicate bugs or security vulnerabilities in smart contracts.
- Predictive Analysis: AI can predict potential failure points in smart contracts based on historical data, enabling developers to proactively address issues before they arise.
- Natural Language Processing (NLP): NLP can assist in understanding and interpreting smart contract code, making it easier for developers to identify logical errors or inconsistencies.
By integrating AI and ML into the debugging process, developers can enhance the reliability of their smart contracts and reduce the risk of costly errors. At Rapid Innovation, we utilize these advanced technologies to streamline our clients' development processes, ensuring they achieve their goals efficiently and effectively.
13.3. Preparing for Future Solana Ecosystem Developments
As the Solana ecosystem continues to evolve, developers must stay informed and prepared for upcoming changes and advancements. This preparation involves understanding the trends and potential developments that could impact the Solana blockchain.
- Stay Updated: Regularly follow Solana's official channels, including their blog and social media, to keep abreast of new features, updates, and community initiatives.
- Engage with the Community: Participate in forums, Discord channels, and developer meetups to share knowledge and learn from others in the ecosystem.
- Experiment with New Tools: As new tools and frameworks emerge, take the time to experiment with them. This hands-on experience will help you adapt to changes more quickly.
- Focus on Scalability: As Solana grows, scalability will be a key concern. Developers should consider how their applications can handle increased traffic and transaction volumes.
- Security Best Practices: With the rise of new technologies, security threats will also evolve. Stay informed about best practices for securing smart contracts and dApps.
By proactively preparing for future developments, developers can ensure that their applications remain relevant and competitive in the rapidly changing Solana ecosystem. Partnering with Rapid Innovation allows clients to navigate these changes with confidence, ensuring they are always at the forefront of innovation and maximizing their return on investment.